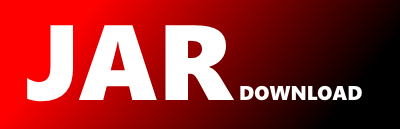
cdc.applic.demos.ExpressionLabelDemo Maven / Gradle / Ivy
package cdc.applic.demos;
import java.awt.Color;
import java.awt.GridBagConstraints;
import java.awt.GridBagLayout;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JTextField;
import javax.swing.SwingUtilities;
import javax.swing.WindowConstants;
import javax.swing.border.EmptyBorder;
import javax.swing.event.DocumentEvent;
import javax.swing.event.DocumentListener;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import cdc.applic.dictionaries.impl.RepositoryImpl;
import cdc.applic.dictionaries.impl.io.RepositoryXml;
import cdc.applic.expressions.Expression;
import cdc.applic.expressions.Formatting;
import cdc.applic.expressions.parsing.Analyzer;
import cdc.applic.expressions.parsing.ParsingEvent;
import cdc.applic.publication.core.formatters.FormattersCatalog;
import cdc.applic.publication.core.formatters.InfixFormatter;
import cdc.applic.publication.core.formatters.io.FormattersCatalogXml;
import cdc.applic.publication.html.Category;
import cdc.applic.publication.html.FontSettings;
import cdc.applic.publication.html.HtmlTextFormattingHandler;
import cdc.applic.publication.html.HtmlTextSettings;
import cdc.applic.publication.html.StringHtmlHandler;
import cdc.applic.ui.swing.ExpressionLabel;
import cdc.ui.swing.GridBagConstraintsBuilder;
import cdc.ui.swing.SwingUtils;
import cdc.util.lang.FailureReaction;
public class ExpressionLabelDemo extends JFrame {
private static final long serialVersionUID = 1L;
private static final Logger LOGGER = LogManager.getLogger(ExpressionLabelDemo.class);
private final JTextField wText = new JTextField();
private final List wLabels = new ArrayList<>();
private final JLabel wHtml = new JLabel();
private RepositoryImpl repository;
private FormattersCatalog catalog;
private final HtmlTextSettings settings;
public ExpressionLabelDemo() {
final RepositoryXml.StAXLoader repositoryLoader = new RepositoryXml.StAXLoader(FailureReaction.WARN);
try {
this.repository = repositoryLoader.load("src/main/resources/repository.xml");
} catch (final IOException e) {
LOGGER.catching(e);
this.repository = null;
}
final FormattersCatalogXml.StAXLoader catalogLoader = new FormattersCatalogXml.StAXLoader(FailureReaction.WARN);
try {
this.catalog = catalogLoader.load("src/main/resources/formatters-catalog.xml");
} catch (final IOException e) {
LOGGER.catching(e);
this.catalog = null;
}
this.settings = HtmlTextSettings.builder()
.set(Category.DEFAULT,
FontSettings.builder()
.face("Arial")
.color("#000000")
.size("+2")
.build())
.set(Category.INFORMAL,
FontSettings.builder()
.color("#FFBBBB")
.build())
.set(Category.PREFIX,
FontSettings.builder()
.color("#FFFF00")
.bold()
.italic()
.build())
.set(Category.ALIAS,
FontSettings.builder()
.color("#FFCC00")
.italic()
.build())
.set(Category.PROPERTY,
FontSettings.builder()
.color("#AAFF00")
.build())
.set(Category.INVALID_NAME,
FontSettings.builder()
.color("#FF4444")
.bold()
.underline()
.build())
.set(Category.VALUE,
FontSettings.builder()
.color("#00BBFF")
.build())
.set(Category.INVALID_VALUE,
FontSettings.builder()
.color("#FF4444")
.bold()
.underline()
.build())
.set(Category.UNKNOWN_VALUE,
FontSettings.builder()
.color("#FF44FF")
.bold()
.underline()
.build())
.set(Category.DELIMITER,
FontSettings.builder()
.color("#AAAAFF")
.build())
.set(Category.OPERATOR,
FontSettings.builder()
.color("#EE77FF")
.build())
.set(Category.SPACE,
FontSettings.builder()
.color("#FFFFFF")
.bold()
.build())
.build();
final JPanel wPanel = new JPanel();
add(wPanel);
wPanel.setLayout(new GridBagLayout());
wText.setColumns(30);
wText.setText("$this is an informal expression$ or R.Rank = 10 or Rank not in {20~30, 40,50} or Rank in {} or Rank in ∅ and not (R.A1 -> (false <-> true) >-< true) & \"A A\" or A or B and C"
+ " and Rank < 10 and Rank <= 10 and Rank !< 10 and Rank !<= 10"
+ " and Rank > 10 and Rank >= 10 and Rank !> 10 and Rank !>= 10");
wText.getDocument().addDocumentListener(new DocumentListener() {
@Override
public void removeUpdate(DocumentEvent e) {
refresh();
}
@Override
public void insertUpdate(DocumentEvent e) {
refresh();
}
@Override
public void changedUpdate(DocumentEvent e) {
refresh();
}
});
wPanel.add(wText,
GridBagConstraintsBuilder.builder()
.gridy(0)
.gridwidth(2)
.weightx(1.0)
.anchor(GridBagConstraints.LINE_START)
.fill(GridBagConstraints.BOTH)
.insets(5, 5, 0, 5)
.build());
wLabels.add(new ExpressionLabel());
wLabels.add(new ExpressionLabel(Formatting.LONG_NARROW));
wLabels.add(new ExpressionLabel(Formatting.LONG_WIDE));
wLabels.add(new ExpressionLabel(Formatting.MATH_NARROW));
wLabels.add(new ExpressionLabel(Formatting.MATH_WIDE));
wLabels.add(new ExpressionLabel(Formatting.SHORT_NARROW));
wLabels.add(new ExpressionLabel(Formatting.SHORT_WIDE));
int y = 1;
for (int index = 0; index < wLabels.size(); index++) {
final ExpressionLabel wLabel = wLabels.get(index);
SwingUtils.setPlainFont(wLabel);
wPanel.add(new JLabel(wLabel.getFormatting() == null ? "As Is: " : Objects.toString(wLabel.getFormatting()) + ": "),
GridBagConstraintsBuilder.builder()
.gridx(0)
.gridy(y)
.weighty(index == wLabels.size() - 1 ? 1.0 : 0.0)
.weighty(0.0)
.weightx(0.0)
.anchor(GridBagConstraints.FIRST_LINE_START)
.insets(5, 5, 0, 0)
.build());
wPanel.add(wLabel,
GridBagConstraintsBuilder.builder()
.gridx(1)
.gridy(y)
.weighty(index == wLabels.size() - 1 ? 1.0 : 0.0)
.weighty(0.0)
.weightx(1.0)
.anchor(GridBagConstraints.FIRST_LINE_START)
.insets(5, 5, 0, 0)
.build());
y++;
}
wPanel.add(new JLabel("HTML"),
GridBagConstraintsBuilder.builder()
.gridx(0)
.gridy(y)
.weighty(1.0)
.weightx(0.0)
.anchor(GridBagConstraints.FIRST_LINE_START)
.insets(5, 5, 0, 0)
.build());
SwingUtils.setPlainFont(wHtml);
wHtml.setOpaque(true);
wHtml.setBackground(new Color(70, 70, 70));
wHtml.setBorder(new EmptyBorder(5, 10, 5, 10));
wPanel.add(wHtml,
GridBagConstraintsBuilder.builder()
.gridx(1)
.gridy(y)
.weighty(1.0)
.weightx(1.0)
.anchor(GridBagConstraints.FIRST_LINE_START)
.insets(5, 5, 0, 0)
.build());
refresh();
}
protected void refresh() {
final Expression expression = new Expression(wText.getText(), false);
for (final ExpressionLabel wLabel : wLabels) {
wLabel.setExpression(expression);
}
final StringHtmlHandler shh = new StringHtmlHandler();
final HtmlTextFormattingHandler htf = new HtmlTextFormattingHandler(settings, shh);
final InfixFormatter iff = new InfixFormatter(repository.getRegistry("/Registry"), catalog);
try {
shh.openTag("html");
shh.openTag("body");
shh.openTag("nobr");
iff.format(expression, htf);
shh.closeTag("nobr");
shh.closeTag("body");
shh.closeTag("html");
wHtml.setText(shh.toString());
wHtml.setToolTipText(null);
LOGGER.info(shh);
} catch (final RuntimeException e) {
wHtml.setText("" + expression.getContent() + "");
wHtml.setToolTipText(e.getMessage());
}
try {
LOGGER.info("expression {}", wText.getText());
final List events = Analyzer.analyze(wText.getText());
for (final ParsingEvent event : events) {
LOGGER.info(event);
}
} catch (final RuntimeException e) {
LOGGER.error(e);
}
}
public static void main(String... args) {
SwingUtilities.invokeLater(() -> {
final ExpressionLabelDemo frame = new ExpressionLabelDemo();
frame.setTitle(ExpressionLabelDemo.class.getSimpleName());
frame.setSize(1200, 400);
frame.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
frame.setVisible(true);
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy