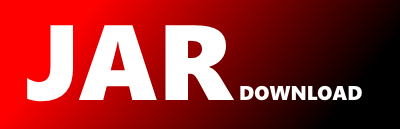
cdc.applic.expressions.ast.AbstractNaryNode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cdc-applic-expressions Show documentation
Show all versions of cdc-applic-expressions Show documentation
Applicabilities Expressions.
The newest version!
package cdc.applic.expressions.ast;
import java.io.PrintStream;
import java.util.Arrays;
import java.util.List;
import cdc.applic.expressions.Formatting;
import cdc.util.debug.Printables;
import cdc.util.lang.Checks;
/**
* Base class of n-ary nodes.
*
* @author Damien Carbonne
*/
public abstract class AbstractNaryNode extends AbstractOperatorNode {
protected static final String NODES = "nodes";
private final Node[] children;
private int hash = 0;
protected AbstractNaryNode(Node... children) {
Checks.isNotNull(children, "children");
if (children.length < 1) {
throw new IllegalArgumentException("No enough children");
}
this.children = children.clone();
}
protected AbstractNaryNode(List extends Node> children) {
this(children.toArray(new Node[children.size()]));
}
@Override
public final int getChildrenCount() {
return children.length;
}
@Override
public final Node getChildAt(int index) {
if (index >= 0 && index < children.length) {
return children[index];
} else {
throw new IllegalArgumentException("Invalid index (" + index + ")");
}
}
public final Node[] getChildren() {
return children;
}
public final List getChildrenAsList() {
return Arrays.asList(children);
}
/**
* Creates an NaryNode with same kind as this node, from an array of nodes.
*
* @param children The children nodes.
* @return An instance of this class combining {@code nodes}.
* @throws IllegalArgumentException When {@code children} is {@code null} or empty.
*/
public abstract AbstractNaryNode create(Node... children);
/**
* Creates an NaryNode with same kind as this node, from an list of nodes.
*
* @param children The children nodes.
* @return An instance of this class combining {@code nodes}.
* @throws IllegalArgumentException When {@code children} is {@code null} or empty.
*/
public abstract AbstractNaryNode create(List children);
@Override
public final int getHeight() {
int max = 0;
for (int index = 0; index < children.length; index++) {
max = Math.max(max, children[index].getHeight());
}
return 1 + max;
}
@Override
public final void print(PrintStream out,
int level) {
Printables.indent(out, level);
out.println(getKind());
for (final Node child : getChildren()) {
child.print(out, level + 1);
}
}
@Override
public final void buildInfix(Formatting formatting,
StringBuilder builder) {
boolean first = true;
boolean previousChildParen = false;
for (final Node child : getChildren()) {
final boolean childParen = needParentheses(child);
if (first) {
first = false;
} else {
getKerning().addSpace(builder, formatting, previousChildParen);
getKerning().addSymbol(builder, formatting);
getKerning().addSpace(builder, formatting, childParen);
}
NodeKerning.addOpenParenthese(builder, childParen);
child.buildInfix(formatting, builder);
NodeKerning.addCloseParenthese(builder, childParen);
previousChildParen = childParen;
}
}
@Override
public R accept(Visitor visitor) {
return visitor.visitNary(this);
}
@Override
public final boolean equals(Object object) {
if (this == object) {
return true;
}
if (object == null || !getClass().equals(object.getClass())) {
return false;
}
final AbstractNaryNode other = (AbstractNaryNode) object;
return Arrays.equals(children, other.children);
}
@Override
public final int hashCode() {
if (hash == 0) {
hash = Arrays.hashCode(children);
}
return hash;
}
@Override
public String toString() {
final StringBuilder builder = new StringBuilder();
builder.append(getKind());
builder.append("(");
for (int index = 0; index < children.length; index++) {
if (index != 0) {
builder.append(",");
}
builder.append(children[index]);
}
builder.append(")");
return builder.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy