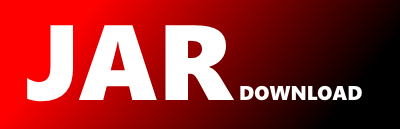
cdc.applic.expressions.ast.AbstractSetNode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cdc-applic-expressions Show documentation
Show all versions of cdc-applic-expressions Show documentation
Applicabilities Expressions.
The newest version!
package cdc.applic.expressions.ast;
import java.io.PrintStream;
import java.util.Objects;
import cdc.applic.expressions.Formatting;
import cdc.applic.expressions.content.SItemSet;
import cdc.applic.expressions.literals.Name;
import cdc.util.debug.Printables;
import cdc.util.lang.Checks;
/**
* Base class of set nodes.
*
* @author Damien Carbonne
*/
public abstract class AbstractSetNode extends AbstractPropertyNode implements SetNode {
private final SItemSet set;
private int hash = 0;
protected AbstractSetNode(Name propertyName,
SItemSet set) {
super(propertyName);
Checks.isNotNull(set, "set");
this.set = set;
}
@Override
public abstract AbstractSetNode negate();
/**
* @return The set of items.
*/
@Override
public final SItemSet getSet() {
return set;
}
/**
* @return The checked set of items.
* It may be {@code null} if set can not be converted to a checked set.
*/
@Override
public final SItemSet getCheckedSet() {
return set.getChecked();
}
/**
* @return The best set of items.
* It is a checked set if possible.
*/
@Override
public final SItemSet getBestSet() {
return set.getBest();
}
@Override
public abstract AbstractSetNode create(Name propertyName,
SItemSet set);
@Override
public final void buildInfix(Formatting formatting,
StringBuilder builder) {
builder.append(getName());
getKerning().addSpace(builder, formatting, false);
getKerning().addSymbol(builder, formatting);
getKerning().addSpace(builder, formatting, true);
// Use getSet() and not getCheckedSet() to preserve input order
builder.append(NodeKerning.toString(getSet(), formatting.getSymbolType()));
}
@Override
public final void print(PrintStream out,
int level) {
Printables.indent(out, level);
out.println(getKind() + " " + getName() + " " + getSet());
}
@Override
public final boolean equals(Object object) {
if (this == object) {
return true;
}
if (object == null || !getClass().equals(object.getClass())) {
return false;
}
final AbstractSetNode other = (AbstractSetNode) object;
return getName().equals(other.getName())
&& getBestSet().equals(other.getBestSet());
}
@Override
public final int hashCode() {
if (hash == 0) {
hash = Objects.hash(getName(),
getBestSet());
}
return hash;
}
@Override
public final String toString() {
return getKind() + "(" + getName()
+ "," + getSet()
+ ")";
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy