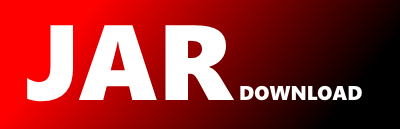
cdc.applic.expressions.ast.NaryOrNode Maven / Gradle / Ivy
Show all versions of cdc-applic-expressions Show documentation
package cdc.applic.expressions.ast;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import cdc.util.lang.Checks;
public final class NaryOrNode extends AbstractNaryNode {
public static final String KIND = "NARY_OR";
public NaryOrNode(Node... children) {
super(children);
}
public NaryOrNode(List extends Node> children) {
super(children);
}
@Override
public String getKind() {
return KIND;
}
@Override
public NodeKerning getKerning() {
return NodeKerning.KERNING_OR;
}
@Override
public final NaryOrNode create(Node... children) {
return new NaryOrNode(children);
}
@Override
public NaryOrNode create(List children) {
return new NaryOrNode(children);
}
/**
* Creates the simplest or node combining a list of nodes.
*
* If {@code nodes} contains 1 node, returns this node.
* If {@code nodes} contains 2 nodes, returns an OrNode.
* If {@code nodes} contains more than 2 nodes, returns an NaryOrNode.
*
* @param nodes The nodes to combine as an Or node.
* @return The simplest or node combining {@code nodes}.
* @throws IllegalArgumentException When {@code nodes} is {@code null} or empty.
*/
public static Node createSimplestOr(List extends Node> nodes) {
Checks.isNotNullOrEmpty(nodes, NODES);
if (nodes.size() == 1) {
return nodes.get(0);
} else if (nodes.size() == 2) {
return new OrNode(nodes.get(0), nodes.get(1));
} else {
return new NaryOrNode(nodes);
}
}
/**
* Creates the simplest or node combining an array of nodes.
*
* If {@code nodes} contains 1 node, returns this node.
* If {@code nodes} contains 2 nodes, returns an OrNode.
* If {@code nodes} contains more than 2 nodes, returns an NaryOrNode.
*
* @param nodes The nodes to combine as an Or node.
* @return The simplest or node combining {@code nodes}.
* @throws IllegalArgumentException When {@code nodes} is {@code null} or empty.
*/
public static Node createSimplestOr(Node... nodes) {
Checks.isNotNullOrEmpty(nodes, NODES);
if (nodes.length == 1) {
return nodes[0];
} else if (nodes.length == 2) {
return new OrNode(nodes[0], nodes[1]);
} else {
return new NaryOrNode(nodes);
}
}
public static Node mergeSimplestOr(Node... nodes) {
Checks.isNotNullOrEmpty(nodes, NODES);
final List children = new ArrayList<>();
for (final Node node : nodes) {
if (node instanceof final NaryOrNode n) {
Collections.addAll(children, n.getChildren());
} else if (node instanceof final OrNode n) {
children.add(n.getAlpha());
children.add(n.getBeta());
} else {
children.add(node);
}
}
return createSimplestOr(children);
}
}