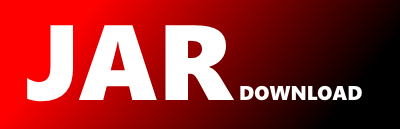
cdc.applic.expressions.ast.NodeKerning Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cdc-applic-expressions Show documentation
Show all versions of cdc-applic-expressions Show documentation
Applicabilities Expressions.
The newest version!
package cdc.applic.expressions.ast;
import cdc.applic.expressions.Formatting;
import cdc.applic.expressions.Spacing;
import cdc.applic.expressions.SymbolType;
import cdc.applic.expressions.content.SItemSet;
import cdc.util.lang.Checks;
/**
* Class containing information (symbols, precedence, ...) on nodes.
*
* @author Damien Carbonne
*/
public final class NodeKerning {
private final String shortSymbol;
private final boolean shortSpace;
private final String longSymbol;
private final boolean longSpace;
private final String mathSymbol;
private final boolean mathSpace;
private final int precedence;
private final boolean ambiguous;
/** Precedence of equivalence operator. */
public static final int PRECEDENCE_EQUIV = 1;
/** Precedence of xor operator. */
public static final int PRECEDENCE_XOR = PRECEDENCE_EQUIV;
/** Precedence of implication operator. */
public static final int PRECEDENCE_IMPL = 2;
/** Precedence of binary, and nary or operator. */
public static final int PRECEDENCE_OR = 3;
/** Precedence of binary, and nary and operator. */
public static final int PRECEDENCE_AND = 4;
/**
* Precedence of property nodes:
*
* - equal, not equal,
*
- in, not in,
*
- less than, not less than,
*
- less or equal, not less or equal,
*
- greater than, not greater than,
*
- greater or equal, not greater or equal
*
*/
public static final int PRECEDENCE_PROPERTY = 5;
/** Precedence of unary not operator. */
public static final int PRECEDENCE_NOT = 6;
/** Precedence of false, true, ref, and other atom nodes */
public static final int PRECEDENCE_ATOM = 7;
public static final NodeKerning KERNING_EQUIV =
new NodeKerning("<->", false, "iff", true, "\u2194", false, PRECEDENCE_EQUIV, true);
public static final NodeKerning KERNING_XOR =
new NodeKerning(">-<", false, "xor", true, "\u21AE", false, PRECEDENCE_XOR, true);
public static final NodeKerning KERNING_IMPL =
new NodeKerning("->", false, "imp", true, "\u2192", false, PRECEDENCE_IMPL, true);
public static final NodeKerning KERNING_OR =
new NodeKerning("|", false, "or", true, "\u2228", false, PRECEDENCE_OR, false);
public static final NodeKerning KERNING_AND =
new NodeKerning("&", false, "and", true, "\u2227", false, PRECEDENCE_AND, false);
public static final NodeKerning KERNING_NOT =
new NodeKerning("!", false, "not", true, "\u00AC", false, PRECEDENCE_NOT, false);
public static final NodeKerning KERNING_EQUAL =
new NodeKerning("=", false, PRECEDENCE_PROPERTY, false);
public static final NodeKerning KERNING_NOT_EQUAL =
new NodeKerning("!=", false, "!=", false, "\u2260", false, PRECEDENCE_PROPERTY, false);
public static final NodeKerning KERNING_IN =
new NodeKerning("<:", false, "in", true, "\u2208", false, PRECEDENCE_PROPERTY, false);
public static final NodeKerning KERNING_NOT_IN =
new NodeKerning("!<:", false, "not in", true, "\u2209", false, PRECEDENCE_PROPERTY, false);
public static final NodeKerning KERNING_LESS =
new NodeKerning("<", false, PRECEDENCE_PROPERTY, false);
public static final NodeKerning KERNING_NOT_LESS =
new NodeKerning("!<", false, "!<", false, "\u226E", false, PRECEDENCE_PROPERTY, false);
public static final NodeKerning KERNING_LESS_OR_EQUAL =
new NodeKerning("<=", false, "<=", false, "\u2264", false, PRECEDENCE_PROPERTY, false);
public static final NodeKerning KERNING_NEITHER_LESS_NOR_EQUAL =
new NodeKerning("!<=", false, "!<=", false, "\u2270", false, PRECEDENCE_PROPERTY, false);
public static final NodeKerning KERNING_GREATER =
new NodeKerning(">", false, PRECEDENCE_PROPERTY, false);
public static final NodeKerning KERNING_NOT_GREATER =
new NodeKerning("!>", false, "!>", false, "\u226F", false, PRECEDENCE_PROPERTY, false);
public static final NodeKerning KERNING_GREATER_OR_EQUAL =
new NodeKerning(">=", false, ">=", false, "\u2265", false, PRECEDENCE_PROPERTY, false);
public static final NodeKerning KERNING_NEITHER_GREATER_NOR_EQUAL =
new NodeKerning("!>=", false, "!>=", false, "\u2271", false, PRECEDENCE_PROPERTY, false);
public static final NodeKerning KERNING_EMPTY_SPACE =
new NodeKerning("{}", true, "{}", true, "\u2205", false, PRECEDENCE_ATOM, false);
public static final NodeKerning KERNING_FALSE =
new NodeKerning("false", true, "false", true, "\u22A5", false, PRECEDENCE_ATOM, false);
public static final NodeKerning KERNING_TRUE =
new NodeKerning("true", true, "true", true, "\u22A4", false, PRECEDENCE_ATOM, false);
public static final NodeKerning KERNING_ATOM =
new NodeKerning(null, false, PRECEDENCE_ATOM, false);
private NodeKerning(String shortSymbol,
boolean shortSpace,
String longSymbol,
boolean longSpace,
String mathSymbol,
boolean mathSpace,
int precedence,
boolean ambiguous) {
this.shortSymbol = shortSymbol;
this.shortSpace = shortSpace;
this.longSymbol = longSymbol;
this.longSpace = longSpace;
this.mathSymbol = mathSymbol;
this.mathSpace = mathSpace;
this.precedence = precedence;
this.ambiguous = ambiguous;
}
private NodeKerning(String symbol,
boolean space,
int precedence,
boolean ambiguous) {
this(symbol, space, symbol, space, symbol, space, precedence, ambiguous);
}
public int getPrecedence() {
return precedence;
}
public boolean isAmbiguous() {
return ambiguous;
}
public String getSymbol(SymbolType type) {
Checks.isNotNull(type, "type");
if (type == SymbolType.SHORT) {
return shortSymbol;
} else if (type == SymbolType.LONG) {
return longSymbol;
} else {
return mathSymbol;
}
}
public boolean needsSpace(SymbolType type) {
Checks.isNotNull(type, "type");
if (type == SymbolType.SHORT) {
return shortSpace;
} else if (type == SymbolType.LONG) {
return longSpace;
} else {
return mathSpace;
}
}
public static void addOpenParenthese(StringBuilder builder,
boolean needed) {
if (needed) {
builder.append('(');
}
}
public static void addCloseParenthese(StringBuilder builder,
boolean needed) {
if (needed) {
builder.append(')');
}
}
/**
* Adds a space if necessary.
*
* This is the case when:
*
* - {@code spacing} is {@link Spacing#WIDE}.
*
- or {@code type} needs space and {@code nextToCompactable} is {@code false}.
*
*
* @param builder The StringBuilder.
* @param formatting The formatting parameters.
* @param nextToCompactable If {@code true} the symbol is next to a compactable literal (that does not need space around it).
*/
public void addSpace(StringBuilder builder,
Formatting formatting,
boolean nextToCompactable) {
Checks.isNotNull(formatting, "formatting");
addSpace(builder, formatting.getSymbolType(), formatting.getSpacing(), nextToCompactable);
}
public void addSpace(StringBuilder builder,
SymbolType type,
Spacing spacing,
boolean nextToCompactable) {
Checks.isNotNull(type, "type");
Checks.isNotNull(spacing, "spacing");
if (spacing == Spacing.WIDE || (!nextToCompactable && needsSpace(type))) {
builder.append(' ');
}
}
public void addSymbol(StringBuilder builder,
Formatting formatting) {
Checks.isNotNull(formatting, "formatting");
builder.append(getSymbol(formatting.getSymbolType()));
}
public static String toString(SItemSet set,
SymbolType symbolType) {
if (symbolType == SymbolType.MATH && set.isEmpty()) {
return "∅";
} else {
return set.toString();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy