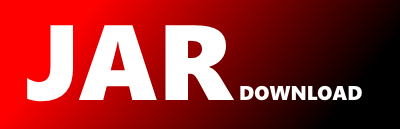
cdc.applic.expressions.ast.Nodes Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cdc-applic-expressions Show documentation
Show all versions of cdc-applic-expressions Show documentation
Applicabilities Expressions.
The newest version!
package cdc.applic.expressions.ast;
import java.util.Collection;
public final class Nodes {
private Nodes() {
}
public static Node notSimplified(Node alpha) {
if (alpha.equals(TrueNode.INSTANCE)) {
return FalseNode.INSTANCE;
} else if (alpha.equals(FalseNode.INSTANCE)) {
return TrueNode.INSTANCE;
} else if (alpha instanceof NotNode) {
return ((NotNode) alpha).getAlpha();
} else {
return new NotNode(alpha);
}
}
public static Node andSimplified(Node alpha,
Node beta) {
if (alpha.equals(FalseNode.INSTANCE) || beta.equals(FalseNode.INSTANCE)) {
return FalseNode.INSTANCE;
} else if (alpha.equals(TrueNode.INSTANCE)) {
return beta;
} else if (beta.equals(TrueNode.INSTANCE)) {
return alpha;
} else {
return new AndNode(alpha, beta);
}
}
public static Node and(Node... alphas) {
if (alphas.length == 1) {
return alphas[0];
} else {
Node n = alphas[0];
for (int index = 1; index < alphas.length; index++) {
n = new AndNode(n, alphas[index]);
}
return n;
}
}
public static Node andSimplified(Node... alphas) {
if (alphas.length == 1) {
return alphas[0];
} else {
int trueCount = 0;
for (final Node alpha : alphas) {
if (alpha.equals(FalseNode.INSTANCE)) {
return FalseNode.INSTANCE;
} else if (alpha.equals(TrueNode.INSTANCE)) {
trueCount++;
}
}
if (trueCount == 0) {
// No true, so we can not do much
return and(alphas);
} else if (trueCount == alphas.length) {
// All true, so result is true
return TrueNode.INSTANCE;
} else {
// Extract non true operands
final Node[] nonTrue = new Node[alphas.length - trueCount];
int index = 0;
for (final Node alpha : alphas) {
if (!alpha.equals(TrueNode.INSTANCE)) {
nonTrue[index] = alpha;
index++;
}
}
return and(nonTrue);
}
}
}
public static Node andSimplified(Collection alphas) {
return andSimplified(alphas.toArray(new Node[alphas.size()]));
}
public static Node orSimplified(Node alpha,
Node beta) {
if (alpha.equals(TrueNode.INSTANCE) || beta.equals(TrueNode.INSTANCE)) {
return TrueNode.INSTANCE;
} else if (alpha.equals(FalseNode.INSTANCE)) {
return beta;
} else if (beta.equals(FalseNode.INSTANCE)) {
return alpha;
} else {
return new OrNode(alpha, beta);
}
}
public static Node or(Node... alphas) {
if (alphas.length == 1) {
return alphas[0];
} else {
Node n = alphas[0];
for (int index = 1; index < alphas.length; index++) {
n = new OrNode(n, alphas[index]);
}
return n;
}
}
public static Node orSimplified(Node... alphas) {
if (alphas.length == 1) {
return alphas[0];
} else {
int falseCount = 0;
for (final Node alpha : alphas) {
if (alpha.equals(TrueNode.INSTANCE)) {
return TrueNode.INSTANCE;
} else if (alpha.equals(FalseNode.INSTANCE)) {
falseCount++;
}
}
if (falseCount == 0) {
// No true, so we can not do much
return or(alphas);
} else if (falseCount == alphas.length) {
// All false, so result is false
return FalseNode.INSTANCE;
} else {
// Extract non false operands
final Node[] nonFalse = new Node[alphas.length - falseCount];
int index = 0;
for (final Node alpha : alphas) {
if (!alpha.equals(FalseNode.INSTANCE)) {
nonFalse[index] = alpha;
index++;
}
}
return or(nonFalse);
}
}
}
public static Node orSimplified(Collection alphas) {
return orSimplified(alphas.toArray(new Node[alphas.size()]));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy