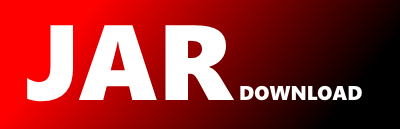
cdc.applic.expressions.ast.Visitor Maven / Gradle / Ivy
Show all versions of cdc-applic-expressions Show documentation
package cdc.applic.expressions.ast;
/**
* Interface implemented by objects that need to be notified during AST
* traversal.
*
* A visit method is defined for each node arity.
* This facilitates the writing of many algorithms whose behavior directly depends on this parameter.
* In addition, each method has a specific name, even if overloading could have been used.
*
* @author D. Carbonne
*
* @param Class of the objects returned by the visit.
*/
public interface Visitor {
/**
* Invoked when a leaf node is visited.
*
* @param node The visited node.
* @return The visit result.
*/
public R visitLeaf(AbstractLeafNode node);
/**
* Invoked when an unary node is visited.
*
* @param node The visited node.
* @return The visit result.
*/
public R visitUnary(AbstractUnaryNode node);
/**
* Invoked when a binary node is visited.
*
* @param node The visited node.
* @return The visit result.
*/
public R visitBinary(AbstractBinaryNode node);
/**
* Invoked when an n-ary node is visited.
*
* @param node The visited node.
* @return The visit result.
*/
public R visitNary(AbstractNaryNode node);
}