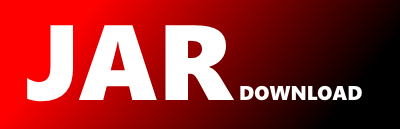
cdc.applic.expressions.ast.visitors.AbstractCollector Maven / Gradle / Ivy
Show all versions of cdc-applic-expressions Show documentation
package cdc.applic.expressions.ast.visitors;
import java.util.Collection;
import java.util.List;
import java.util.Set;
import cdc.util.lang.Checks;
/**
* Base class of collectors.
*
* They collect information and accumulate it in a collection.
*
* @author Damien Carbonne
*
* @param Type of the collected information.
*/
public class AbstractCollector extends AbstractAnalyzer {
protected final Collection collection;
protected AbstractCollector(Collection collection) {
Checks.isNotNull(collection, "collection");
this.collection = collection;
}
protected void add(T element) {
collection.add(element);
}
protected void addAll(Collection extends T> elements) {
collection.addAll(elements);
}
/**
* @return The collection.
*/
public Collection getCollection() {
return collection;
}
/**
* @return The collection as a set.
*/
public Set getSet() {
return (Set) collection;
}
/**
* @return The collection as a list.
*/
public List getList() {
return (List) collection;
}
}