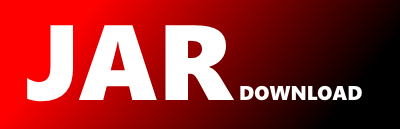
cdc.applic.expressions.ast.visitors.EliminateTrueFalse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cdc-applic-expressions Show documentation
Show all versions of cdc-applic-expressions Show documentation
Applicabilities Expressions.
The newest version!
package cdc.applic.expressions.ast.visitors;
import java.util.ArrayList;
import java.util.List;
import cdc.applic.expressions.ast.AbstractBinaryNode;
import cdc.applic.expressions.ast.AbstractNaryNode;
import cdc.applic.expressions.ast.AbstractUnaryNode;
import cdc.applic.expressions.ast.FalseNode;
import cdc.applic.expressions.ast.NaryAndNode;
import cdc.applic.expressions.ast.NaryOrNode;
import cdc.applic.expressions.ast.Node;
import cdc.applic.expressions.ast.OrNode;
import cdc.applic.expressions.ast.TrueNode;
/**
* A Converter that applies the following rewriting rules:
*
* - ¬⊤ ≡ ⊥
*
- ¬⊥ ≡ ⊤
*
- ⊤∧β ≡ β∧⊤ ≡ β
*
- ⊥∧β ≡ β∧⊥ ≡ ⊥
*
- ⊤∨β ≡ β∨⊤ ≡ ⊤
*
- ⊥∨β ≡ β∨⊥ ≡ β
*
*
* @author Damien Carbonne
*/
public final class EliminateTrueFalse extends AbstractConverter {
private static final EliminateTrueFalse CONVERTER = new EliminateTrueFalse();
private EliminateTrueFalse() {
}
public static Node execute(Node node) {
return node.accept(CONVERTER);
}
@Override
public Node visitUnary(AbstractUnaryNode node) {
final Node alpha = node.getAlpha().accept(this);
if (alpha instanceof TrueNode) {
return FalseNode.INSTANCE;
} else if (alpha instanceof FalseNode) {
return TrueNode.INSTANCE;
} else {
return node.create(alpha);
}
}
@Override
public Node visitBinary(AbstractBinaryNode node) {
final Node alpha = node.getAlpha().accept(this);
if (alpha instanceof TrueNode) {
if (node instanceof OrNode) {
// true or beta
return TrueNode.INSTANCE;
} else {
// true and beta
return node.getBeta().accept(this);
}
} else if (alpha instanceof FalseNode) {
if (node instanceof OrNode) {
// false or beta
return node.getBeta().accept(this);
} else {
// false and beta
return FalseNode.INSTANCE;
}
} else {
final Node beta = node.getBeta().accept(this);
if (beta instanceof TrueNode) {
if (node instanceof OrNode) {
// alpha or true
return TrueNode.INSTANCE;
} else {
// alpha and true
return alpha;
}
} else if (beta instanceof FalseNode) {
if (node instanceof OrNode) {
// alpha or false
return alpha;
} else {
// alpha and false
return FalseNode.INSTANCE;
}
} else {
return node.create(alpha, beta);
}
}
}
@Override
public Node visitNary(AbstractNaryNode node) {
// new children
final List children = new ArrayList<>();
for (Node child : node.getChildren()) {
child = child.accept(this);
if (child instanceof TrueNode) {
if (node instanceof NaryOrNode) {
// ... or true or ...
return TrueNode.INSTANCE;
}
// ... and true and ...
} else if (child instanceof FalseNode) {
if (node instanceof NaryAndNode) {
// ... and false and ...
return FalseNode.INSTANCE;
}
// ... or false or ...
} else {
children.add(child);
}
}
if (children.isEmpty()) {
if (node instanceof NaryAndNode) {
return TrueNode.INSTANCE;
} else {
return FalseNode.INSTANCE;
}
} else if (children.size() == 1) {
return children.get(0);
} else if (node instanceof NaryOrNode) {
return new NaryOrNode(children);
} else {
return new NaryAndNode(children);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy