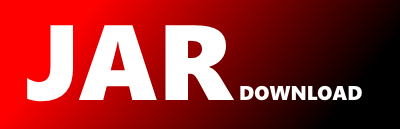
cdc.applic.expressions.checks.ApplicIssue Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cdc-applic-expressions Show documentation
Show all versions of cdc-applic-expressions Show documentation
Applicabilities Expressions.
The newest version!
package cdc.applic.expressions.checks;
import java.util.List;
import java.util.Objects;
import cdc.issues.Issue;
import cdc.issues.Params;
import cdc.issues.locations.Location;
import cdc.util.lang.Checks;
import cdc.util.lang.CollectionUtils;
/**
* Description of an issue.
*
* @author Damien Carbonne
*/
public class ApplicIssue extends Issue {
public static final String DOMAIN = ApplicRuleUtils.DOMAIN;
private final Throwable cause;
protected ApplicIssue(Builder> builder) {
super(builder);
this.cause = builder.cause;
}
public ApplicIssue(ApplicIssueType type,
String project,
List extends Location> locations,
String description,
Throwable cause) {
super(DOMAIN,
type,
Params.NO_PARAMS,
project,
locations,
null,
type.getSeverity(),
description,
Params.NO_PARAMS);
this.cause = cause;
}
public ApplicIssue(ApplicIssueType type,
String project,
List extends Location> locations,
String description) {
this(type,
project,
locations,
description,
null);
}
public ApplicIssue(ApplicIssueType type,
String project,
Location location,
String description,
Throwable cause) {
this(type,
project,
CollectionUtils.toList(location),
description,
cause);
}
public ApplicIssue(ApplicIssueType type,
String project,
Location location,
String description) {
this(type,
project,
location,
description,
null);
}
public ApplicIssueType getIssueType() {
return getName(ApplicIssueType.class);
}
public Throwable getCause() {
return cause;
}
@Override
public int hashCode() {
return 31 * super.hashCode()
+ Objects.hash(cause);
}
@Override
public boolean equals(Object object) {
if (!super.equals(object)) {
return false;
}
final ApplicIssue other = (ApplicIssue) object;
return Objects.equals(cause, other.cause);
}
public static ApplicIssueType getMostSevereType(List issues) {
Checks.isNotNull(issues, "issues");
ApplicIssueType max = ApplicIssueType.NO_ISSUE;
for (final ApplicIssue issue : issues) {
max = ApplicIssueType.mostSevere(max, issue.getIssueType());
}
return max;
}
public static Builder> builder() {
return new Builder<>();
}
public static class Builder> extends Issue.Builder {
private Throwable cause;
protected Builder() {
super();
}
public B cause(Throwable cause) {
this.cause = cause;
return self();
}
protected B fix() {
domain(DOMAIN);
return self();
}
@Override
public ApplicIssue build() {
return new ApplicIssue(fix());
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy