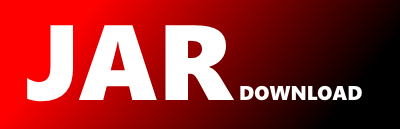
cdc.applic.expressions.content.BooleanValue Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cdc-applic-expressions Show documentation
Show all versions of cdc-applic-expressions Show documentation
Applicabilities Expressions.
The newest version!
package cdc.applic.expressions.content;
import java.util.List;
import cdc.applic.expressions.literals.LiteralUtils;
import cdc.graphs.PartialOrderPosition;
import cdc.graphs.PartiallyComparable;
/**
* Class representing a single boolean value.
*
* @author Damien Carbonne
*/
public final class BooleanValue implements BooleanSItem, Value, Comparable, PartiallyComparable {
private final boolean value;
public static final BooleanValue FALSE = new BooleanValue(false);
public static final BooleanValue TRUE = new BooleanValue(true);
public static final List FALSE_TRUE = List.of(FALSE, TRUE);
private BooleanValue(boolean value) {
this.value = value;
}
/**
* Creates a {@link BooleanValue} from a {@code boolean}.
*
* @param value The value.
* @return A new {@link BooleanValue} from {@code value}.
*/
public static BooleanValue of(boolean value) {
return value ? TRUE : FALSE;
}
/**
* Creates a {@link BooleanValue} from a its String representation.
*
* @param literal The boolean literal.
* @return A new {@link BooleanValue} from {@code literal}.
* @throws IllegalArgumentException When {@code literal} can not be recognized as a {@code boolean}.
*/
public static BooleanValue of(String literal) {
if (!LiteralUtils.isBooleanLiteral(literal)) {
throw new IllegalArgumentException("Illegal boolean literal (" + literal + ")");
}
return of(Boolean.parseBoolean(literal));
}
public BooleanValue negate() {
return this == TRUE ? FALSE : TRUE;
}
@Override
public String getNonEscapedLiteral() {
return value ? "true" : "false";
}
@Override
public String getProtectedLiteral() {
return getNonEscapedLiteral();
}
public boolean getValue() {
return value;
}
@Override
public int compareTo(BooleanValue o) {
if (this == o) {
return 0;
}
if (o == null) {
return 1;
} else {
return this == FALSE ? -1 : 1;
}
}
@Override
public PartialOrderPosition partialCompareTo(BooleanValue o) {
return PartiallyComparable.partialCompare(this, o);
}
@Override
public boolean equals(Object object) {
// Booleans are cached and there are only 2 values.
// So we can use this simple approach
return this == object;
}
@Override
public int hashCode() {
return Boolean.hashCode(value);
}
@Override
public String toString() {
return getProtectedLiteral();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy