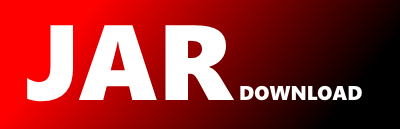
cdc.applic.expressions.content.RealRange Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cdc-applic-expressions Show documentation
Show all versions of cdc-applic-expressions Show documentation
Applicabilities Expressions.
The newest version!
package cdc.applic.expressions.content;
import java.util.Comparator;
/**
* Class representing a real range.
*
* @author Damien Carbonne
*/
public final class RealRange extends AbstractRange implements RealSItem {
public static final Comparator MIN_COMPARATOR = minComparator();
public static final Comparator MAX_COMPARATOR = maxComparator();
private RealRange(RealValue value) {
super(value);
}
private RealRange(RealValue min,
RealValue max) {
super(min, max);
}
private RealRange(double value) {
this(RealValue.of(value));
}
private RealRange(double min,
double max) {
this(RealValue.of(min),
RealValue.of(max));
}
@Override
protected RealRange self() {
return this;
}
@Override
public RealDomain getDomain() {
return RealDomain.INSTANCE;
}
/**
* Creates a {@link RealRange} from a single {@link RealValue}.
*
* @param value The value.
* @return A new {@link RealRange} from {@code value}.
*/
public static RealRange of(RealValue value) {
return new RealRange(value);
}
/**
* Creates a {@link RealRange} from 2 {@link RealValue RealValues}.
*
* @param min The min value.
* @param max The max value.
* @return A new {@link RealRange} from {@code min} and {@code max}.
*/
public static RealRange of(RealValue min,
RealValue max) {
return new RealRange(min, max);
}
/**
* Creates a {@link RealRange} from a single {@code double}.
*
* @param value The value.
* @return A new {@link RealRange} from {@code value}.
*/
public static RealRange of(double value) {
return new RealRange(value);
}
/**
* Creates a {@link RealRange} from 2 {@code doubles}.
*
* @param min The min value.
* @param max The max value.
* @return A new {@link RealRange} from {@code min} and {@code max}.
*/
public static RealRange of(double min,
double max) {
return new RealRange(min, max);
}
public boolean contains(double value) {
return containsValue(RealValue.of(value));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy