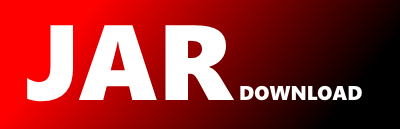
cdc.applic.expressions.content.RealSet Maven / Gradle / Ivy
Show all versions of cdc-applic-expressions Show documentation
package cdc.applic.expressions.content;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Comparator;
import java.util.List;
import cdc.applic.expressions.SyntacticException;
import cdc.applic.expressions.parsing.SItemsParsing;
import cdc.graphs.PartialOrderPosition;
import cdc.util.lang.Checks;
/**
* Implementation of {@link SItemSet} containing {@link RealSItem}s.
*
* Order of definition of SItems is lost.
* Duplicates are removed.
*
* @author Damien Carbonne
*/
public final class RealSet extends AbstractRangeSet {
public static final RealSet EMPTY = new RealSet();
public static final Comparator LEXICOGRAPHIC_COMPARATOR = lexicographicComparator();
private RealSet(List ranges) {
super(RealDomain.INSTANCE,
ranges);
}
private RealSet() {
super(RealDomain.INSTANCE);
}
private RealSet(Collection extends RealSItem> items) {
super(RealDomain.INSTANCE,
toRanges(items));
}
private RealSet(String content) {
this(SItemsParsing.toRealRanges(content));
}
@SafeVarargs
private RealSet(RealSItem... items) {
super(RealDomain.INSTANCE,
toRanges(items));
}
private static List toRanges(Collection extends RealSItem> items) {
final List result = new ArrayList<>();
for (final RealSItem item : items) {
if (item instanceof RealValue) {
result.add(RealRange.of((RealValue) item));
} else {
result.add((RealRange) item);
}
}
return result;
}
private static List toRanges(RealSItem... items) {
final List result = new ArrayList<>();
for (final RealSItem item : items) {
if (item instanceof RealValue) {
result.add(RealRange.of((RealValue) item));
} else {
result.add((RealRange) item);
}
}
return result;
}
@Override
protected RealSet create(List ranges) {
return new RealSet(ranges);
}
@Override
protected RealSet adapt(SItemSet set) {
return convert(set);
}
@Override
public RealSet empty() {
return EMPTY;
}
@Override
protected RealSet self() {
return this;
}
/**
* Converts an {@link SItemSet} set to a {@link RealSet}.
*
* @param set The set.
* @return The conversion of {@code set} to an {@link RealSet}.
* @throws IllegalArgumentException When {@code set} can not be converted to an {@link RealSet}.
*/
public static RealSet convert(SItemSet set) {
Checks.isNotNull(set, "set");
if (set instanceof RealSet) {
return (RealSet) set;
} else if (set.isEmpty()) {
return EMPTY;
} else if (set.isCompliantWith(RealSet.class)) {
// Should not throw ClassCastException
return new RealSet(convert(set.getItems(), RealSItem.class));
} else {
throw new IllegalArgumentException("Can not convert this set to " + RealSet.class.getSimpleName());
}
}
/**
* Creates a {@link RealSet} from the String representation of its content.
*
* @param content The content.
* @return A new instance of {@link RealSet}.
* @throws SyntacticException When {@code content} can not be parsed as a {@link RealSet}.
*/
public static RealSet of(String content) {
return new RealSet(content);
}
/**
* Creates a {@link RealSet} from an array of {@link RealSItem RealSItems}.
*
* @param items The items.
* @return A new instance of {@link RealSet}.
* @throws IllegalArgumentException When an item is {@code null}.
*/
public static RealSet of(RealSItem... items) {
return new RealSet(items);
}
/**
* Creates a {@link RealSet} from a collection of {@link RealSItem RealSItems}.
*
* @param items The items.
* @return A new instance of {@link RealSet}.
* @throws IllegalArgumentException When an item is {@code null}.
*/
public static RealSet of(Collection extends RealSItem> items) {
return new RealSet(items);
}
/**
* Creates a {@link RealSet} from one {@link RealSItem}.
*
* @param item The item.
* @return A new instance of {@link RealSet}.
* @throws IllegalArgumentException When {@code value} is {@code null}.
*/
public static RealSet of(RealSItem item) {
return new RealSet(item);
}
public boolean contains(double value) {
return contains(RealValue.of(value));
}
public RealSet union(double value) {
return union(RealValue.of(value));
}
public RealSet intersection(double value) {
return intersection(RealValue.of(value));
}
public RealSet remove(double value) {
return remove(RealValue.of(value));
}
public static RealSet of(RealValue value,
PartialOrderPosition position) {
return AbstractRangeSet.toSet(RealDomain.INSTANCE,
EMPTY,
RealSet::of,
value,
position);
}
}