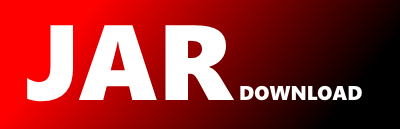
cdc.applic.expressions.content.SItemSet Maven / Gradle / Ivy
Show all versions of cdc-applic-expressions Show documentation
package cdc.applic.expressions.content;
import java.util.Comparator;
import java.util.List;
import cdc.applic.expressions.IllegalOperationException;
/**
* Interface implemented by set of {@link SItem}s.
*
* @author Damien Carbonne
*/
public interface SItemSet {
public static final String ITEMS_SEPARATOR = ",";
public Comparator COMPARATOR = SItemSetUtils::compare;
/**
* @return The checked version of this set, if possible.
* An empty {@link UncheckedSet} is a valid result.
* If this set is non empty and can not be converted to
* a checked set, {@code null} is returned.
*/
public SItemSet getChecked();
/**
* @return The checked version of this set if possible,
* this set otherwise.
*/
public SItemSet getBest();
/**
* @return A BooleanSet view of this set.
* @throws IllegalArgumentException When this set can not be converted to a BooleanSet.
*/
public BooleanSet toBooleanSet();
/**
* @return An IntegerSet view of this set.
* @throws IllegalArgumentException When this set can not be converted to an IntegerSet.
*/
public IntegerSet toIntegerSet();
/**
* @return A RealSet view of this set.
* @throws IllegalArgumentException When this set can not be converted to an RealSet.
*/
public RealSet toRealSet();
/**
* @return A StringSet view of this set.
* @throws IllegalArgumentException When this set can not be converted to a StringSet.
*/
public StringSet toStringSet();
/**
* @return {@code true} if this set is empty.
*/
public boolean isEmpty();
/**
* @return {@code true} if this set contains ranges.
*/
public boolean containsRanges();
/**
* @return {@code true} if this set is a singleton.
*/
public boolean isSingleton();
/**
* @return The singleton value if this set is a singleton.
* @throws IllegalOperationException When this set is not a singleton.
*/
public Value getSingletonValue();
/**
* Returns {@code true} if this set is compliant with a type of set.
*
* @param cls The tested set class.
* @return {@code true} if this set is compliant with a set whose class is {@code cls}.
*/
public boolean isCompliantWith(Class extends SItemSet> cls);
/**
* Returns {@code true} if this set is valid.
*
* This is the case for checked sets.
*
* @return {@code true} if this set is valid.
*/
public boolean isValid();
/**
* @return {@code true} if this set is checked.
* All empty sets are checked.
*/
public boolean isChecked();
/**
* @return A list of items contained in this set.
*/
public List extends SItem> getItems();
/**
* @return The string representation of the content of this set.
*/
public String getContent();
/**
* Returns {@code true} if this set contains an item.
*
* @param item The SItem.
* @return {@code true} if this set contains {@code item}.
*/
public boolean contains(SItem item);
/**
* Returns {@code true} if this set contains an item set.
*
* @param set The SItemSet.
* @return {@code true} if this set contains {@code set}.
*/
public boolean contains(SItemSet set);
/**
* Returns the union of this set with an item.
*
* @param item The SItem.
* @return the union of this set with {@code item}.
*/
public SItemSet union(SItem item);
/**
* Returns the union of this set with an item set.
*
* @param set The SItemSet.
* @return the union of this set with {@code set}.
*/
public SItemSet union(SItemSet set);
/**
* Returns the intersection of this set with an item.
*
* @param item The SItem.
* @return the intersection of this set with {@code item}.
*/
public SItemSet intersection(SItem item);
/**
* Returns the intersection of this set with an item set.
*
* @param set The SItemSet.
* @return the intersection of this set with {@code set}.
*/
public SItemSet intersection(SItemSet set);
/**
* Returns the content of this set excluding an item.
*
* @param item The SItem.
* @return The content of this set excluding {@code item}.
*/
public SItemSet remove(SItem item);
/**
* Returns the content of this set excluding an item set.
*
* @param set The SItemSet.
* @return The content of this set excluding {@code set}.
*/
public SItemSet remove(SItemSet set);
/**
* Returns {@code true} if this set has the same content as another set.
*
* @param set The SItemSet.
* @return {@code true} if this set has the same content as {@code set}.
*/
public boolean hasSameContentAs(SItemSet set);
}