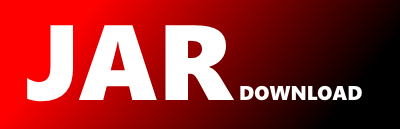
cdc.applic.expressions.content.ValueUtils Maven / Gradle / Ivy
Show all versions of cdc-applic-expressions Show documentation
package cdc.applic.expressions.content;
import cdc.applic.expressions.literals.EscapingUtils;
import cdc.applic.expressions.literals.LiteralUtils;
public final class ValueUtils {
private ValueUtils() {
}
/**
* Creates a Value corresponding to a literal.
*
* WARNING: literal MUST be escaped if necessary.
*
* @param literal The literal, escaped if necessary.
* @return The Value corresponding to literal.
* @throws IllegalArgumentException When literal is invalid.
*/
public static Value create(String literal) {
if (EscapingUtils.isValidDoubleQuotesEscapedText(literal)) {
return StringValue.of(EscapingUtils.unescapeDoubleQuotes(literal), false);
} else if (LiteralUtils.isBooleanLiteral(literal)) {
return BooleanValue.of(literal);
} else if (LiteralUtils.isIntegerLiteral(literal)) {
return IntegerValue.of(literal);
} else if (LiteralUtils.isRealLiteral(literal)) {
return RealValue.of(literal);
} else if (LiteralUtils.isRestrictedStringLiteral(literal)) {
return StringValue.of(literal, false);
} else {
throw new IllegalArgumentException("Illegal literal ('" + literal + "')");
}
}
/**
* Compares 2 Values.
*
* If {@code v1} and {@code v2} have the same type, the corresponding comparator is used.
* Otherwise, their string representations are compared.
*
* @param v1 The 1st value.
* @param v2 The 2nd value.
* @return The comparison of {@code v1} and {@code v2}.
*/
public static int compare(Value v1,
Value v2) {
if (v1 == v2) {
return 0;
}
if (v1 == null || v2 == null) {
return v1 == null ? -1 : 1;
}
if (v1.getClass().equals(v2.getClass())) {
if (v1 instanceof BooleanValue) {
return ((BooleanValue) v1).compareTo((BooleanValue) v2);
} else if (v1 instanceof StringValue) {
return ((StringValue) v1).compareTo((StringValue) v2);
} else if (v1 instanceof IntegerValue) {
return ((IntegerValue) v1).compareTo((IntegerValue) v2);
} else {
return ((RealValue) v1).compareTo((RealValue) v2);
}
} else {
return v1.toString().compareTo(v2.toString());
}
}
}