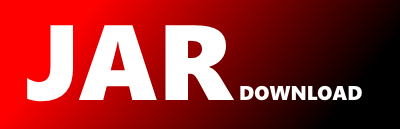
cdc.applic.expressions.parsing.Token Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cdc-applic-expressions Show documentation
Show all versions of cdc-applic-expressions Show documentation
Applicabilities Expressions.
The newest version!
package cdc.applic.expressions.parsing;
import java.util.Objects;
import cdc.applic.expressions.literals.EscapingUtils;
import cdc.util.lang.Checks;
public final class Token {
private final TokenType type;
private final String expression;
private final int beginIndex;
private final int endIndex;
public Token(TokenType type,
String expression,
int beginIndex,
int endIndex) {
Checks.isNotNull(type, "type");
this.type = type;
this.expression = expression;
this.beginIndex = beginIndex;
this.endIndex = endIndex;
}
public TokenType getType() {
return type;
}
public String getExpression() {
return expression;
}
public int getBeginIndex() {
return beginIndex;
}
public int getEndIndex() {
return endIndex;
}
/**
* @return The part of expression in [beginIndex,endIndex[.
*/
public String getText() {
if (expression == null) {
return null;
} else if (beginIndex >= expression.length() || endIndex <= beginIndex) {
return "";
} else {
return expression.substring(getBeginIndex(), getEndIndex());
}
}
/**
* @return The unescaped text. Escape may be '"' or '$'.
*/
public String getUnescapedText() {
final String text = getText();
if (getType() == TokenType.DOUBLE_QUOTES_TEXT) {
return EscapingUtils.unescapeDoubleQuotes(text);
} else if (getType() == TokenType.DOLLAR_TEXT) {
return EscapingUtils.unescapeDollars(text);
} else {
return text;
}
}
@Override
public int hashCode() {
return Objects.hash(type,
beginIndex,
endIndex,
expression);
}
@Override
public boolean equals(Object object) {
if (this == object) {
return true;
}
if (!(object instanceof Token)) {
return false;
}
final Token other = (Token) object;
return type == other.type
&& beginIndex == other.beginIndex
&& endIndex == other.endIndex
&& Objects.equals(expression, other.expression);
}
@Override
public String toString() {
final StringBuilder builder = new StringBuilder();
builder.append("[");
builder.append(getType());
builder.append(", '");
if (getExpression() != null) {
builder.append(getText());
}
builder.append("', at ");
builder.append(getBeginIndex());
builder.append("]");
return builder.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy