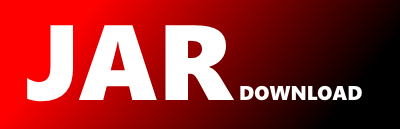
cdc.applic.expressions.ExpressionPool Maven / Gradle / Ivy
Show all versions of cdc-applic-expressions Show documentation
package cdc.applic.expressions;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
/**
* Simple Expression pool that can be used to share Expressions.
*
* An Expression is functionally immutable.
*
* @author Damien Carbonne
*/
public class ExpressionPool {
private final Map map = new HashMap<>();
public void clear() {
map.clear();
}
/**
* @return The set of contents to which an expression is associated.
*/
public Set getExpressionContents() {
return map.keySet();
}
/**
* @param content The content.
* @return The shared Expression associated to {@code content}.
*/
public Expression get(String content) {
return map.computeIfAbsent(content, Expression::new);
}
/**
* @param expression The Expression.
* @return The shared Expression associated to {@code expression}.
*/
public Expression get(Expression expression) {
return get(expression.getContent());
}
}