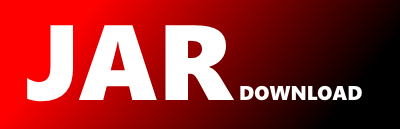
cdc.applic.expressions.LexicalException Maven / Gradle / Ivy
Show all versions of cdc-applic-expressions Show documentation
package cdc.applic.expressions;
import cdc.util.lang.Checks;
/**
* Exception raised to indicate that an expression is lexically invalid.
*
* Information on the location of the problem is embedded.
*
* Error is found during tokenizing.
*
* @author Damien Carbonne
*/
public class LexicalException extends ApplicException {
private static final long serialVersionUID = -1139923600878287349L;
private final Detail detail;
private final String info;
private final String expression;
private final int beginIndex;
private final int endIndex;
/**
* Enumeration of exception details.
*
* @author Damien Carbonne
*/
public enum Detail {
/** During tokenization, an invalid (real or integer) number is found. */
INVALID_NUMBER,
/** During tokenization, a (real or integer) number is not followed by a boundary. */
MISSING_BOUNDARY,
/** During tokenization, closing quotes are missing. */
MISSING_CLOSING_QUOTES,
/** During tokenization, an empty escaped text is found. */
EMPTY_ESCAPED_TEXT
}
public LexicalException(Detail detail,
String info,
String expression,
int beginIndex,
int endIndex) {
super(info + ": '" + expression + "'", null);
Checks.isNotNull(expression, "expression");
Checks.isTrue(beginIndex >= 0, "");
this.info = info;
this.detail = detail;
this.expression = expression;
this.beginIndex = beginIndex;
this.endIndex = endIndex;
}
public String getInfo() {
return info;
}
/**
* @return The exception detail.
*/
public Detail getDetail() {
return detail;
}
/**
* @return The expression text.
*/
public String getExpression() {
return expression;
}
/**
* @return The begin index (inclusive) in {@code expression} of the error location.
*/
public int getBeginIndex() {
return beginIndex;
}
/**
* @return The end index (exclusive) in {@code expression} of the error location.
*/
public int getEndIndex() {
return endIndex;
}
public String getSlice() {
final StringBuilder builder = new StringBuilder();
final int begin = getBeginIndex();
final int end = getEndIndex() >= 0 ? getEndIndex() : getExpression().length();
for (int index = 0; index < begin; index++) {
builder.append(" ");
}
for (int index = begin; index < end; index++) {
builder.append("^");
}
return builder.toString();
}
public String getFullMessage() {
final StringBuilder builder = new StringBuilder();
builder.append(getDetail());
builder.append(" ");
builder.append(getInfo());
builder.append("\n");
builder.append(" '" + getExpression() + "'\n");
builder.append(" " + getSlice());
return builder.toString();
}
}