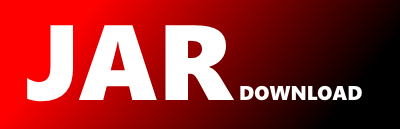
cdc.applic.expressions.ast.AbstractValueNode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cdc-applic-expressions Show documentation
Show all versions of cdc-applic-expressions Show documentation
Applicabilities Expressions.
package cdc.applic.expressions.ast;
import java.io.PrintStream;
import java.util.Objects;
import cdc.applic.expressions.Formatting;
import cdc.applic.expressions.content.Value;
import cdc.applic.expressions.literals.Name;
import cdc.util.debug.Printables;
import cdc.util.lang.Checks;
/**
* Base class of value nodes.
*
* @author Damien Carbonne
*/
public abstract class AbstractValueNode extends AbstractPropertyNode implements ValueNode {
private final Value value;
private int hash = 0;
protected AbstractValueNode(Name propertyName,
Value value) {
super(propertyName);
Checks.isNotNull(value, "value");
this.value = value;
}
@Override
public abstract AbstractValueNode negate();
@Override
public abstract AbstractValueNode create(Name propertyName,
Value value);
@Override
public final Value getValue() {
return value;
}
@Override
public final void buildInfix(Formatting formatting,
StringBuilder builder) {
builder.append(getName());
getKerning().addSpace(builder, formatting, false);
getKerning().addSymbol(builder, formatting);
getKerning().addSpace(builder, formatting, false);
builder.append(getValue().getProtectedLiteral());
}
@Override
public final void print(PrintStream out,
int level) {
Printables.indent(out, level);
out.println(getKind() + " " + getName() + " " + getValue());
}
@Override
public final int hashCode() {
if (hash == 0) {
hash = Objects.hash(getName(),
getValue());
}
return hash;
}
@Override
public final boolean equals(Object object) {
if (this == object) {
return true;
}
if (object == null || !getClass().equals(object.getClass())) {
return false;
}
final AbstractValueNode other = (AbstractValueNode) object;
return getName().equals(other.getName())
&& getValue().equals(other.getValue());
}
@Override
public final String toString() {
return getKind() + "(" + getName()
+ "," + getValue().getProtectedLiteral()
+ ")";
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy