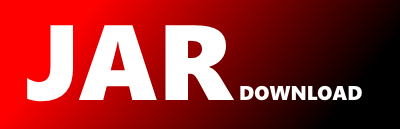
cdc.applic.expressions.checks.CheckedData Maven / Gradle / Ivy
Show all versions of cdc-applic-expressions Show documentation
package cdc.applic.expressions.checks;
import cdc.applic.expressions.Expression;
import cdc.applic.expressions.LexicalException;
import cdc.applic.expressions.SyntacticException;
import cdc.applic.expressions.ast.Node;
import cdc.util.lang.Checks;
/**
* Wrapper of data that can be checked by a {@link Checker}.
*
* If possible and meaningful, a context should be passed.
* This gives better information about location of an issues.
*
* @author Damien Carbonne
*/
public final class CheckedData {
private final String context;
private final Expression expression;
private final Node node;
/**
* Creates an Expression wrapper.
*
* @param context The expression context.
* @param expression The expression.
* @throws IllegalArgumentException When {@code expression} is {@code null}.
*/
public CheckedData(String context,
Expression expression) {
this.context = context;
this.expression = Checks.isNotNull(expression, "expression");
this.node = null;
}
/**
* Creates a context-less Expression wrapper.
*
* @param expression The expression.
* @throws IllegalArgumentException When {@code expression} is {@code null}.
*/
public CheckedData(Expression expression) {
this(null, expression);
}
/**
* Creates an Expression wrapper.
*
* @param context The expression context.
* @param expression The expression.
*/
public CheckedData(String context,
String expression) {
this.context = context;
this.expression = new Expression(expression, false);
this.node = null;
}
/**
* Creates a context-less Expression wrapper.
*
* @param expression The expression.
*/
public CheckedData(String expression) {
this(null, expression);
}
/**
* Creates a Node wrapper.
*
* @param context The node context.
* @param node The node.
* @throws IllegalArgumentException When {@code node} is {@code null}.
*/
public CheckedData(String context,
Node node) {
this.context = context;
this.expression = null;
this.node = Checks.isNotNull(node, "node");
}
/**
* Creates a context-less node wrapper.
*
* @param node The node.
* @throws IllegalArgumentException When {@code node} is {@code null}.
*/
public CheckedData(Node node) {
this(null, node);
}
/**
* @return The expression if {@link CheckedData#CheckedData(Expression)} was used,
* {@code null} otherwise.
*/
public Expression getExpression() {
return expression;
}
/**
* @return The context of the wrapped expression / node.
*/
public String getContext() {
return context;
}
/**
* @return The node if {@link CheckedData#CheckedData(Node)} was used,
* the root node of the expression otherwise.
* @throws LexicalException When this was constructed with an invalid Expression.
* @throws SyntacticException When this was constructed with an invalid Expression.
*/
public Node getNode() {
return node == null ? expression.getRootNode() : node;
}
public String getContent() {
if (expression == null) {
return node.compress();
} else {
return expression.getContent();
}
}
@Override
public String toString() {
return "[" + context + " " + getContent() + "]";
}
}