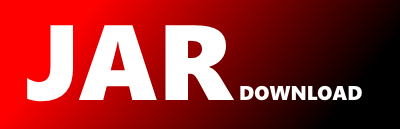
cdc.applic.expressions.checks.Checker Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cdc-applic-expressions Show documentation
Show all versions of cdc-applic-expressions Show documentation
Applicabilities Expressions.
package cdc.applic.expressions.checks;
import java.util.ArrayList;
import java.util.List;
import cdc.applic.expressions.ApplicException;
import cdc.applic.expressions.Expression;
import cdc.applic.expressions.ast.Node;
import cdc.issues.Diagnosis;
import cdc.issues.IssuesHandler;
/**
* Base interface of checkers.
*
* @author Damien Carbonne
*/
public interface Checker {
/**
* @return The name of the rule checked by this checker.
*/
public String getRuleName();
/**
* Returns {@code true} if a {@link CheckedData} is compliant with the checked rule.
*
* @param data The {@link CheckedData}.
* @return {@code true} if {@code data} is compliant with the rule checked by this checker.
* @throws IllegalArgumentException When {@code data} is {@code null}.
*/
public boolean isCompliant(CheckedData data);
/**
* Returns {@code true} if an {@link Expression} is compliant with the checked rule.
*
* @param expression The {@link Expression}.
* @return {@code true} if {@code expression} is compliant with the rule checked by this checker.
*/
public default boolean isCompliant(Expression expression) {
return isCompliant(new CheckedData(null, expression));
}
/**
* Returns {@code true} if an expression is compliant with the checked rule.
*
* @param expression The expression.
* @return {@code true} if {@code expression} is compliant with the rule checked by this checker.
*/
public default boolean isCompliant(String expression) {
return isCompliant(new CheckedData(null, expression));
}
/**
* Returns {@code true} if a {@link Node} is compliant with the checked rule.
*
* @param node The {@link Node}.
* @return {@code true} if {@code node} is compliant with the rule checked by this checker.
* @throws IllegalArgumentException When {@code node} is {@code null}.
*/
public default boolean isCompliant(Node node) {
return isCompliant(new CheckedData(null, node));
}
/**
* Checks the compliance of a {@link CheckedData} and throws an exception if it is not the case.
*
* @param data The {@link CheckedData}.
* @throws IllegalArgumentException When {@code data} is {@code null}.
* @throws ApplicException When a compliance issue is detected.
*/
public void checkCompliance(CheckedData data);
/**
* Checks the compliance of an {@link Expression} and throws an exception if it is not the case.
*
* @param expression The {@link Expression}.
* @throws IllegalArgumentException When {@code expression} is {@code null}.
* @throws ApplicException When a compliance issue is detected.
*/
public default void checkCompliance(Expression expression) {
checkCompliance(new CheckedData(expression));
}
/**
* Checks the compliance of an expression and throws an exception if it is not the case.
*
* @param expression The expression.
* @throws ApplicException When a compliance issue is detected.
*/
public default void checkCompliance(String expression) {
checkCompliance(new Expression(expression, false));
}
/**
* Checks a {@link CheckedData} and adds detected issues to a list.
*
* @param data The {@link CheckedData}.
* @param issues The list of issues that must be filled.
* @throws IllegalArgumentException When {@code data} or {@code issues} is {@code null}.
*/
public void check(CheckedData data,
List issues);
/**
* Checks an {@link Expression} and adds detected issues to a list.
*
* @param expression The {@link Expression}.
* @param issues The list of issues that must be filled.
* @throws IllegalArgumentException When {@code expression} or {@code issues} is {@code null}.
*/
public default void check(Expression expression,
List issues) {
check(new CheckedData(expression),
issues);
}
/**
* Checks an expression and adds detected issues to a list.
*
* @param expression The expression.
* @param issues The list of issues that must be filled.
* @throws IllegalArgumentException When {@code issues} is {@code null}.
*/
public default void check(String expression,
List issues) {
check(new CheckedData(expression),
issues);
}
/**
* Checks a {@link Node} and adds detected issues to a list.
*
* @param node The {@link Node}.
* @param issues The list of issues that must be filled.
* @throws IllegalArgumentException When {@code node} or {@code issues} is {@code null}.
*/
public default void check(Node node,
List issues) {
check(new CheckedData(node),
issues);
}
/**
* Checks a {@link CheckedData} and builds a {@link Diagnosis} with detected issues.
*
* @param data The {@link CheckedData}.
* @return A {@link Diagnosis} with issues detected by this checker in {@code data}.
* @throws IllegalArgumentException When {@code data} is {@code null}.
*/
public default Diagnosis check(CheckedData data) {
final List issues = new ArrayList<>();
check(data, issues);
return Diagnosis.create(issues);
}
/**
* Checks an {@link Expression} and builds a {@link Diagnosis} with detected issues.
*
* @param expression The {@link Expression}.
* @return A {@link Diagnosis} with issues detected by this checker in {@code expression}.
* @throws IllegalArgumentException When {@code expression} is {@code null}.
*/
public default Diagnosis check(Expression expression) {
return check(new CheckedData(expression));
}
/**
* Checks an expression and builds a {@link Diagnosis} with detected issues.
*
* @param expression The expression.
* @return A {@link Diagnosis} with issues detected by this checker in {@code expression}.
*/
public default Diagnosis check(String expression) {
return check(new Expression(expression, false));
}
public default void check(Expression expression,
IssuesHandler handler) {
final List issues = new ArrayList<>();
check(expression, issues);
handler.issues(issues);
}
public static String wrap(Object object) {
return "'" + object + "'";
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy