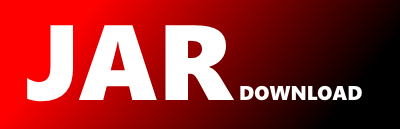
cdc.applic.expressions.content.AbstractSItemSet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cdc-applic-expressions Show documentation
Show all versions of cdc-applic-expressions Show documentation
Applicabilities Expressions.
package cdc.applic.expressions.content;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import cdc.applic.expressions.IllegalOperationException;
import cdc.util.lang.Checks;
public abstract class AbstractSItemSet implements SItemSet {
protected static final String ITEM = "item";
protected static final String SET = "set";
protected static final String VALUE = "value";
protected final IllegalArgumentException nonSupportedItem(SItem item) {
return new IllegalArgumentException("Non supported item " + item.getClass().getSimpleName() + " (" + item + ") by "
+ getClass().getSimpleName() + " " + this);
}
protected final IllegalArgumentException nonSupportedSet(SItemSet set) {
return new IllegalArgumentException("Non supported set (" + set + ") by " + getClass().getSimpleName() + " " + this);
}
/**
* Checks that this set is a singleton.
*
* @throws IllegalOperationException When this set is not a singleton.
*/
protected final void checkIsSingleton() {
if (!isSingleton()) {
throw new IllegalOperationException("This set is not a singleton " + this);
}
}
/**
* Converts a collection of {@link SItem SItems} to a list with narrower class.
*
* @param The target type.
* @param items The collection of items.
* @param targetClass The target class.
* @return The conversion of {@code items} to a List view containing {@code targetClass} items.
* @throws ClassCastException When {@code items} contains items that can not be converted.
*/
protected static List convert(Collection extends SItem> items,
Class targetClass) {
final List result = new ArrayList<>();
for (final SItem item : items) {
result.add(targetClass.cast(item));
}
return result;
}
@Override
public final boolean isCompliantWith(Class extends SItemSet> cls) {
if (isEmpty() || getClass().equals(cls)) {
return true;
} else {
for (final SItem item : getItems()) {
if (!item.isCompliantWith(cls)) {
return false;
}
}
return true;
}
}
@Override
public final BooleanSet toBooleanSet() {
return BooleanSet.convert(this);
}
@Override
public final IntegerSet toIntegerSet() {
return IntegerSet.convert(this);
}
@Override
public final RealSet toRealSet() {
return RealSet.convert(this);
}
@Override
public final StringSet toStringSet() {
return StringSet.convert(this);
}
@Override
public String getContent() {
final StringBuilder builder = new StringBuilder();
boolean first = true;
for (final SItem item : getItems()) {
if (!first) {
builder.append(ITEMS_SEPARATOR);
}
builder.append(item.getProtectedLiteral());
first = false;
}
return builder.toString();
}
@Override
public final boolean contains(SItemSet set) {
Checks.isNotNull(set, SET);
if (set.isEmpty()) {
return true;
} else {
for (final SItem item : set.getItems()) {
if (!contains(item)) {
return false;
}
}
return true;
}
}
@Override
public boolean hasSameContentAs(SItemSet set) {
Checks.isNotNull(set, SET);
return this.contains(set) && set.contains(this);
}
@Override
public String toString() {
final StringBuilder builder = new StringBuilder();
builder.append("{");
builder.append(getContent());
builder.append("}");
return builder.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy