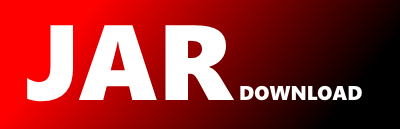
cdc.applic.expressions.content.CheckedSet Maven / Gradle / Ivy
Show all versions of cdc-applic-expressions Show documentation
package cdc.applic.expressions.content;
/**
* Interface of sets that are checked.
*
* Their content is homogeneous.
*
* @author Damien Carbonne
* @param The set type.
* @param The value type.
*/
public interface CheckedSet, V extends Value> extends SItemSet {
@Override
public S getChecked();
@Override
public S getBest();
@Override
public V getSingletonValue();
/**
* Returns {@code true} if this set contains a value.
*
* @param value The value.
* @return {@code true} if this set contains {@code value}.
*/
public boolean contains(V value);
/**
* Returns {@code true} if this set contains another set.
*
* @param set The set.
* @return {@code true} if this set contains {@code set}.
*/
public boolean contains(S set);
/**
* Returns the union of this set with a value.
*
* @param value The value.
* @return the union of this set with {@code value}.
*/
public S union(V value);
/**
* Returns the union of this set with another set.
*
* @param set The set.
* @return the union of this set with {@code set}.
*/
public S union(S set);
/**
* Returns the intersection of this set with a value.
*
* @param value The value.
* @return the intersection of this set with {@code value}.
*/
public S intersection(V value);
/**
* Returns the intersection of this set with another set.
*
* @param set The set.
* @return the intersection of this set with {@code set}.
*/
public S intersection(S set);
/**
* Returns the content of this set excluding a value.
*
* @param value The value.
* @return The content of this set excluding {@code value}.
*/
public S remove(V value);
/**
* Returns the content of this set excluding another set.
*
* @param set The set.
* @return The content of this set excluding {@code set}.
*/
public S remove(S set);
}