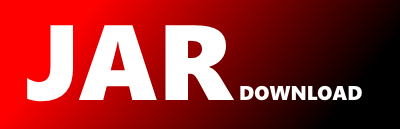
cdc.applic.expressions.parsing.ComparisonOperator Maven / Gradle / Ivy
Show all versions of cdc-applic-expressions Show documentation
package cdc.applic.expressions.parsing;
/**
* Enumeration of comparison operators.
*
* They match value nodes.
*
* @author Damien Carbonne
*/
public enum ComparisonOperator {
/** π = υ */
EQUAL,
/** π ≠ υ */
NOT_EQUAL,
/** π < υ */
LESS,
/** π ≮ υ */
NOT_LESS,
/** π ≤ υ */
LESS_OR_EQUAL,
/** π ≰ υ */
NEITHER_LESS_NOR_EQUAL,
/** π > υ */
GREATER,
/** π ≯ υ */
NOT_GREATER,
/** π ≥ υ */
GREATER_OR_EQUAL,
/** π ≱ υ */
NEITHER_GREATER_NOR_EQUAL;
private static final ComparisonOperator[] OPPOSITE = {
NOT_EQUAL,
EQUAL,
NOT_LESS,
LESS,
NEITHER_LESS_NOR_EQUAL,
LESS_OR_EQUAL,
NOT_GREATER,
GREATER,
NEITHER_GREATER_NOR_EQUAL,
GREATER_OR_EQUAL
};
/**
* @return {@code true} when this operator is negative.
*/
public boolean isNegative() {
return this == NOT_EQUAL
|| this == NOT_LESS
|| this == NOT_GREATER
|| this == NEITHER_LESS_NOR_EQUAL
|| this == NEITHER_GREATER_NOR_EQUAL;
}
/**
* @return The negation of this operator.
*/
public ComparisonOperator negate() {
return OPPOSITE[this.ordinal()];
}
/**
* @return {@code true} if the set of values designated by this operator includes the reference value.
*/
public boolean includesEqual() {
return this == EQUAL
|| this == NOT_LESS
|| this == LESS_OR_EQUAL
|| this == NOT_GREATER
|| this == GREATER_OR_EQUAL;
}
/**
* @return {@code true} if the set of values designated by this operator includes values that are less than the reference value.
*/
public boolean includesLess() {
return this == NOT_EQUAL
|| this == LESS
|| this == LESS_OR_EQUAL
|| this == NOT_GREATER
|| this == NEITHER_GREATER_NOR_EQUAL;
}
/**
* @return {@code true} if the set of values designated by this operator includes values that are greater than the reference value.
*/
public boolean includesGreater() {
return this == NOT_EQUAL
|| this == NOT_LESS
|| this == NEITHER_LESS_NOR_EQUAL
|| this == GREATER
|| this == GREATER_OR_EQUAL;
}
/**
* @return {@code true} if the set of values designated by this operator includes values that are not related to the reference value.
*/
public boolean includesUnrelated() {
return this == NOT_EQUAL
|| this == NOT_LESS
|| this == NEITHER_LESS_NOR_EQUAL
|| this == NOT_GREATER
|| this == NEITHER_GREATER_NOR_EQUAL;
}
}