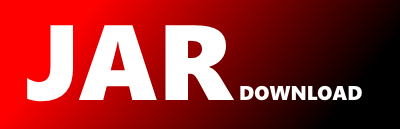
cdc.applic.pclauses.POrClause Maven / Gradle / Ivy
package cdc.applic.pclauses;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import cdc.applic.expressions.ast.AbstractUnaryNode;
import cdc.applic.expressions.ast.FalseNode;
import cdc.applic.expressions.ast.LeafNode;
import cdc.applic.expressions.ast.NaryOrNode;
import cdc.applic.expressions.ast.Node;
import cdc.applic.expressions.ast.NotNode;
import cdc.applic.expressions.ast.ParsingNode;
import cdc.applic.expressions.ast.RefNode;
import cdc.applic.expressions.ast.TrueNode;
public final class POrClause extends PClause {
public static final POrClause TRUE = of(TrueNode.INSTANCE);
public static final POrClause FALSE = of(FalseNode.INSTANCE);
private POrClause(List nodes) {
super(filter(nodes));
}
public static POrClause of(List nodes) {
return new POrClause(nodes);
}
public static POrClause of(Node... nodes) {
return of(Arrays.asList(nodes));
}
private static List filter(List nodes) {
final List result = new ArrayList<>();
for (final Node node : nodes) {
if (node instanceof NotNode) {
final AbstractUnaryNode unode = (AbstractUnaryNode) node;
if (unode.getAlpha() instanceof RefNode) {
result.add(node);
} else {
throw new IllegalArgumentException("Can not add negation of " + unode.getAlpha().getKind() + " nodes");
}
} else if (node instanceof LeafNode && node instanceof ParsingNode) {
result.add(node);
} else {
throw new IllegalArgumentException("Can not add " + node.getKind() + " nodes");
}
}
return result;
}
@Override
public Connective getConnective() {
return Connective.OR;
}
@Override
public Node toNode() {
if (nodes.isEmpty()) {
return FalseNode.INSTANCE;
} else {
return NaryOrNode.createSimplestOr(nodes);
}
}
@Override
public String toString() {
final StringBuilder builder = new StringBuilder();
if (nodes.isEmpty()) {
builder.append(FalseNode.INSTANCE);
} else if (nodes.size() == 1) {
builder.append(nodes.get(0));
} else {
builder.append(getConnective());
builder.append('(');
boolean first = true;
for (final Node node : getNodes()) {
if (!first) {
builder.append(',');
}
builder.append(node);
first = false;
}
builder.append(')');
}
return builder.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy