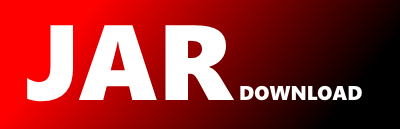
cdc.applic.renaming.RenamingProfile Maven / Gradle / Ivy
package cdc.applic.renaming;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import cdc.applic.expressions.literals.Name;
import cdc.applic.expressions.literals.SName;
/**
* List of renaming steps.
*
* @author Damien Carbonne
*/
public class RenamingProfile {
private final List steps;
private final Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy