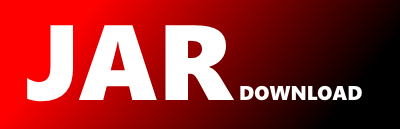
cdc.bench.support.Formatting Maven / Gradle / Ivy
package cdc.bench.support;
import java.text.SimpleDateFormat;
import java.util.Calendar;
public class Formatting {
public static final int KILO = 1000;
public static final int MEGA = KILO * KILO;
public static final int GIGA = KILO * MEGA;
public static final int KILI = 1024;
public static final int MEGI = KILI * KILI;
public static final int GIGI = KILI * MEGI;
private static final SimpleDateFormat SDF = new SimpleDateFormat("yyyy/MM/dd HH:mm:ss,S");
public static String formatSizeInKiB(long bytes) {
return String.format("%.3f", (double) bytes / (double) KILI);
}
public static String formatSize(long bytes) {
if (bytes >= GIGI) {
return String.format("%.3f GiB", (double) bytes / (double) GIGI);
} else if (bytes >= MEGI) {
return String.format("%.3f MiB", (double) bytes / (double) MEGI);
} else if (bytes >= KILI) {
return String.format("%.3f KiB", (double) bytes / (double) KILI);
} else {
return String.format("%d B", bytes);
}
}
public static String formatRateInKiBPers(long bytes,
long nanos) {
final double bytesPerSec = bytes * GIGA / nanos;
return formatRateInKiBPers(bytesPerSec);
}
public static String formatRateInKiBPers(double bytesPerSec) {
return String.format("%.3f", bytesPerSec / KILI);
}
public static String formatRate(double rate,
double unit) {
return String.format("%.3f", rate / unit);
}
public static String formatRate(long bytes,
long nanos) {
final double bytesPerSec = bytes * GIGA / nanos;
if (bytesPerSec >= GIGI) {
return String.format("%.3f GiB/s", bytesPerSec / GIGI);
} else if (bytesPerSec >= MEGI) {
return String.format("%.3f MiB/s", bytesPerSec / MEGI);
} else if (bytesPerSec >= KILI) {
return String.format("%.3f KiB/s", bytesPerSec / KILI);
} else {
return String.format("%d B/s", (int) bytesPerSec);
}
}
public static String formatNanosInms(long nanos) {
final double seconds = (double) nanos / (double) GIGA;
return formatSecondsInms(seconds);
}
public static String formatSecondsInms(double seconds) {
final double millis = seconds * KILO;
return String.format("%.3f", millis);
}
public static String getCurrentTime() {
final Calendar cal = Calendar.getInstance();
cal.getTime();
return SDF.format(cal.getTime());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy