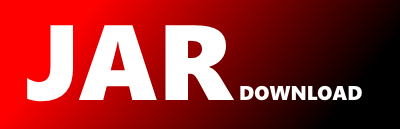
cdc.bench.support.checks.IntegerChecker Maven / Gradle / Ivy
package cdc.bench.support.checks;
public class IntegerChecker implements Checker {
private final int min;
private final int max;
public static final IntegerChecker INT_INSTANCE = new IntegerChecker();
public static final IntegerChecker NAT_INSTANCE = new IntegerChecker(0, Integer.MAX_VALUE);
public static final IntegerChecker POS_INSTANCE = new IntegerChecker(1, Integer.MAX_VALUE);
public IntegerChecker() {
this.min = Integer.MIN_VALUE;
this.max = Integer.MAX_VALUE;
}
public IntegerChecker(int min,
int max) {
this.min = min;
this.max = max;
}
@Override
public CheckResult check(String value) {
try {
final int x = Integer.parseInt(value);
if (x < min) {
return new CheckResult(CheckResult.Status.ERROR, "Too small integer: " + x);
} else if (x > max) {
return new CheckResult(CheckResult.Status.ERROR, "Too large integer: " + x);
} else {
return new CheckResult(CheckResult.Status.OK, value);
}
} catch (final NumberFormatException e) {
return new CheckResult(CheckResult.Status.ERROR, "Invalid integer literal: " + value);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy