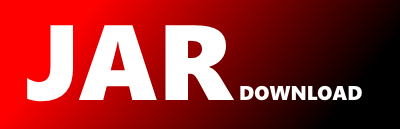
cdc.gv.GvWriterContext Maven / Gradle / Ivy
package cdc.gv;
/**
* Class used to handle writer status stack.
*
* @author Damien Carbonne
*
*/
final class GvWriterContext {
/**
* Enumeration of possible context statuses.
*
*/
public enum Status {
/** Stream is open and waiting graph creation. */
IN_OPEN_STREAM,
/**
* A graph has been started. A subgraph or a cluster can be started. The
* graph can be closed.
*/
IN_GRAPH,
/**
* A subgraph has been started. A subgraph or a cluster can be started.
* The subgraph can be closed.
*/
IN_SUBGRAPH,
/**
* A cluster has been started. A subgraph or a cluster can be started.
* The
* cluster can be closed.
*/
IN_CLUSTER,
/** Graph has been closed. */
IN_CLOSE_STREAM
}
/**
* Context status. May be changed when a context is reused.
*/
private Status status;
/**
* Context name. May be changed when a context is reused.
*/
private String name;
/** Parent context. */
private final GvWriterContext parent;
/** Child context. */
private GvWriterContext child = null;
/** Context level. */
private final int level;
/**
* Create a context.
*
* @param status Context status.
* @param parent Parent context.
*/
private GvWriterContext(Status status,
GvWriterContext parent) {
this.status = status;
this.parent = parent;
if (parent == null) {
this.level = -1;
} else {
this.level = parent.level + 1;
parent.child = this;
}
}
/**
* Create a root (no parent) context with IN_OPEN_STREAM status.
*/
GvWriterContext() {
this(Status.IN_OPEN_STREAM, null);
}
/**
* Return the context level.
*
* @return The context level.
*/
int getLevel() {
return level;
}
/**
* Push a child context to this context. If necessary, a new context is
* created. If possible, unused contexts are reused.
*
* @param status Status of the added context.
* @return The added context.
*/
GvWriterContext pushContext(Status status) {
if (child == null) {
// Create a new child context
return new GvWriterContext(status, this);
} else {
// Reuse existing child context
child.setStatus(status);
return child;
}
}
/**
* Pop this context and return its parent context. The popped context is
* kept
* in memory for future reuse.
*
* @return The parent context.
*/
GvWriterContext popContext() {
status = null;
return parent;
}
/**
* Set the context status. Should rarely be used.
*
* @param status The status to set.
*/
void setStatus(Status status) {
this.status = status;
}
/**
* Return the status of this context.
*
* @return The status of this context.
*/
Status getStatus() {
return status;
}
/**
* Set the name of this context. Meaningful when status is IN_GRAPH,
* IN_SUBGRAPH or IN_CLUSTER.
*
* @param name The name to set.
*/
void setName(String name) {
this.name = name;
}
String getName() {
return name;
}
@Override
public String toString() {
final StringBuilder builder = new StringBuilder();
builder.append(status);
if (name != null) {
builder.append(" ").append(name);
}
return builder.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy