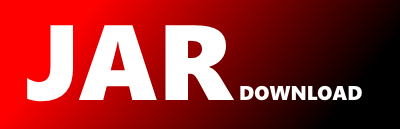
cdc.gv.support.GvSupport Maven / Gradle / Ivy
package cdc.gv.support;
public final class GvSupport {
private GvSupport() {
}
/**
* Returns true when a character is a letter (a-zA-Z).
*
* @param c The character.
* @return True when c is a letter.
*/
private static boolean isLetter(char c) {
return 'a' <= c && c <= 'z'
|| 'A' <= c && c <= 'Z';
}
/**
* Returns true when a character is a digit (0-9).
*
* @param c The character.
* @return True when c is a digit.
*/
private static boolean isDigit(char c) {
return '0' <= c && c <= '9';
}
/**
* Returns true when a character is basic (a-zA-Z0-9_)
*
* @param c The character.
* @return True when c is a basic character.
*/
private static boolean isBasicChar(char c) {
return isLetter(c) || isDigit(c) || c == '_';
}
/**
* Returns true when a string is basic.
*
* It s not null, not empty, all its characters are basic and it does not start by a digit.
*
* @param s The string.
* @return True when s is a basic string.
*/
public static boolean isBasic(String s) {
// We do not accept extended ASCII (128-255)
if (s == null || s.isEmpty()) {
return false;
}
for (int index = 0; index < s.length(); index++) {
final char c = s.charAt(index);
if (!isBasicChar(c)) {
return false;
}
}
return !isDigit(s.charAt(0));
}
/**
* Returns true when a string is numeral.
*
* ([-])[0-9]+([.][0-9]+)
*
* @param s The string
* @return true when s is numeral.
*/
public static boolean isNumeral(String s) {
if (s == null || s.isEmpty()) {
return false;
}
int index = 0;
if (s.charAt(0) == '-') {
index = 1;
}
while (index < s.length() && isDigit(s.charAt(index))) {
index++;
}
if (index == s.length()) {
if (index == 1) {
return s.charAt(0) != '-';
} else {
return true;
}
} else if (s.charAt(index) == '.') {
index++;
if (index == s.length()) {
return false;
} else {
while (index < s.length() && isDigit(s.charAt(index))) {
index++;
}
return index == s.length();
}
} else {
return false;
}
}
public static boolean isDoubleQuoted(String s) {
if (s == null || s.length() < 3) {
return false;
}
if (s.charAt(0) != '"' || s.charAt(s.length() - 1) != '"') {
return false;
}
for (int index = 1; index < s.length() - 1; index++) {
final char c = s.charAt(index);
if ((c == '"' || c == '\n') && s.charAt(index - 1) != '\\') {
return false;
}
}
return true;
}
public static String protect(String s) {
final StringBuilder builder = new StringBuilder();
builder.append('"');
for (int index = 0; index < s.length(); index++) {
final char c = s.charAt(index);
if (c == '"' || c == '\\') {
builder.append('\\');
}
builder.append(c);
}
builder.append('"');
return builder.toString();
}
public static String toValidId(String id) {
// An ID is one of the following:
// - any string of alphabetic ([a-zA-Z\200-\377]) characters,
// underscores ('_') or digits ([0-9]), not beginning with a digit
// - a numeral [-]?(.[0-9]+ | [0-9]+(.[0-9]*)? )
// - any double-quoted string ("...") possibly containing escaped quotes (\")
// - an HTML string (<...>).
if (id == null || id.isEmpty()) {
throw new IllegalArgumentException("Invalid " + (id == null ? "null" : "empty") + " string for id.");
} else if (isBasic(id) || isNumeral(id) || isDoubleQuoted(id)) {
return id;
} else {
return protect(id);
}
}
/**
* Prepends "cluster_" to a string.
*
* @param name The cluster name.
* @return The id that must be used for cluster named name.
*/
public static String toClusterId(String name) {
return toValidId("cluster_" + name);
}
}