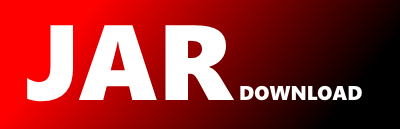
cdc.gv.demo.GvBrewerColorsDemo Maven / Gradle / Ivy
package cdc.gv.demo;
import java.io.File;
import java.io.IOException;
import cdc.gv.GvWriter;
import cdc.gv.atts.GvClusterAttributes;
import cdc.gv.atts.GvEdgeAttributes;
import cdc.gv.atts.GvEdgeStyle;
import cdc.gv.atts.GvGraphAttributes;
import cdc.gv.atts.GvLabelLoc;
import cdc.gv.atts.GvNodeAttributes;
import cdc.gv.atts.GvNodeShape;
import cdc.gv.atts.GvNodeStyle;
import cdc.gv.colors.GvBrewerColors;
import cdc.gv.colors.GvColor;
import cdc.gv.colors.GvX11Colors;
import cdc.gv.colors.GvBrewerColors.SchemeFamily;
import cdc.gv.support.GvSupport;
import cdc.gv.tools.GvEngine;
import cdc.gv.tools.GvFormat;
import cdc.gv.tools.GvToAny;
import cdc.util.files.Files;
/**
* Generate a GraphViz sample file with all Brewer colors.
*
* @author Damien Carbonne
*
*/
public final class GvBrewerColorsDemo {
private GvBrewerColorsDemo() {
}
private static String getId(SchemeFamily family,
int familyIndex,
int colorIndex) {
return family.name() + familyIndex + "-" + colorIndex;
}
private static String getId(SchemeFamily family,
int familyIndex) {
return family.name() + familyIndex;
}
public static void main(String[] args) {
final String odir = "target";
Files.mkdir(odir);
final String name = odir + "/" + GvBrewerColorsDemo.class.getSimpleName() + ".gv";
try (final GvWriter writer = new GvWriter(name)) {
final GvGraphAttributes gatts = new GvGraphAttributes();
gatts.setLabel("Brewer Colors");
gatts.setFontSize(100.0);
gatts.setFontName("Arial");
gatts.setLabelLoc(GvLabelLoc.TOP);
writer.beginGraph("test-brewer-colors", true, gatts);
// Create a cluster for each family
for (final SchemeFamily family : SchemeFamily.values()) {
final GvClusterAttributes fatts = new GvClusterAttributes();
fatts.setColor(GvX11Colors.BLACK);
fatts.setLabel(family.name());
fatts.setFontSize(20.0);
writer.beginCluster(family.name(), fatts);
// Create a cluster for each index in the family
for (int familyIndex = SchemeFamily.MIN_INDEX; familyIndex <= family.getMaxIndex(); familyIndex++) {
final GvClusterAttributes catts = new GvClusterAttributes();
catts.setColor(GvX11Colors.BLACK);
catts.setLabel(family + " " + familyIndex);
writer.beginCluster(getId(family, familyIndex), catts);
// Create a node for each color
for (int colorIndex = 1; colorIndex <= familyIndex; colorIndex++) {
final GvColor color = GvBrewerColors.create(family, familyIndex, colorIndex);
final GvNodeAttributes natts = new GvNodeAttributes();
natts.setColor(GvX11Colors.BLACK);
natts.setFillColor(color);
natts.setShape(GvNodeShape.BOX);
natts.setStyle(GvNodeStyle.FILLED);
natts.setLabel(colorIndex + "");
writer.addNode(getId(family, familyIndex, colorIndex), natts);
}
writer.endCluster();
if (familyIndex > SchemeFamily.MIN_INDEX) {
final GvEdgeAttributes eatts = new GvEdgeAttributes();
eatts.setColor(GvX11Colors.BLACK);
eatts.setLogicalHead(GvSupport.toClusterId(getId(family, familyIndex - 1)));
eatts.setLogicalTail(GvSupport.toClusterId(getId(family, familyIndex)));
eatts.setStyle(GvEdgeStyle.INVIS);
writer.addEdge(getId(family, familyIndex - 1, 1), getId(family, familyIndex, 1), eatts);
}
}
writer.endCluster();
}
writer.endGraph();
writer.flush();
final GvToAny.MainArgs margs = new GvToAny.MainArgs();
margs.setEnabled(GvToAny.MainArgs.Feature.VERBOSE, true);
margs.input = new File(name);
margs.outputDir = new File(odir);
margs.engine = GvEngine.DOT;
margs.formats.add(GvFormat.PDF);
GvToAny.execute(margs);
System.out.println("Done");
} catch (final IOException e) {
e.printStackTrace();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy