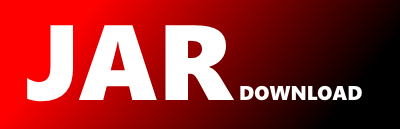
cdc.gv.demo.GvHtmlLabelDemo Maven / Gradle / Ivy
package cdc.gv.demo;
import java.io.File;
import java.io.IOException;
import cdc.gv.GvWriter;
import cdc.gv.atts.GvGraphAttributes;
import cdc.gv.atts.GvNodeAttributes;
import cdc.gv.atts.GvNodeShape;
import cdc.gv.atts.GvNodeStyle;
import cdc.gv.atts.GvRankDir;
import cdc.gv.colors.GvX11Colors;
import cdc.gv.labels.GvCellAttributes;
import cdc.gv.labels.GvHtmlLabel;
import cdc.gv.labels.GvStyle;
import cdc.gv.labels.GvTableAttributes;
import cdc.gv.tools.GvEngine;
import cdc.gv.tools.GvFormat;
import cdc.gv.tools.GvToAny;
import cdc.util.files.Files;
public final class GvHtmlLabelDemo {
private GvHtmlLabelDemo() {
}
public static void main(String[] args) throws IOException {
final String odir = "target";
final String output = odir + "/" + GvHtmlLabelDemo.class.getSimpleName() + ".gv";
Files.mkdir(odir);
final GvWriter writer = new GvWriter(output);
try {
writer.addComment("Beginning of graph");
final GvGraphAttributes gatts = new GvGraphAttributes();
gatts.setRatio(1.0).setMaximumSize(10.0, 10.0).setRankDir(GvRankDir.LR).setNodeSep(0.0);
writer.beginGraph("test-html-labels", true, gatts);
writer.println();
{
final GvHtmlLabel label = new GvHtmlLabel();
final GvTableAttributes tatts = new GvTableAttributes();
tatts.setBgColor(GvX11Colors.ORANGE, GvX11Colors.CYAN);
tatts.setBorder(10);
tatts.setCellSpacing(20);
tatts.setCellPadding(10);
tatts.setColor(GvX11Colors.GREEN);
tatts.setStyle(GvStyle.ROUNDED);
label.beginTable(tatts);
label.beginRow();
final GvCellAttributes catts = new GvCellAttributes();
catts.setBgColor(GvX11Colors.RED, GvX11Colors.YELLOW);
catts.setColor(GvX11Colors.BLUE);
catts.setBorder(5);
catts.setStyle(GvStyle.ROUNDED);
label.beginCell(catts);
label.addText("Table/Row[1]/Cell[1]");
label.endCell();
label.beginCell(catts);
label.addText("Table/Row[1]/Cell[2]");
label.endCell();
label.endRow();
label.beginRow();
catts.setColSpan(2);
label.beginCell(catts);
label.addText("Table/Row[2]/Cell[1..2]");
label.endCell();
label.endRow();
label.beginRow();
label.beginCell(catts);
label.beginTable(tatts);
label.beginRow();
label.beginCell(catts);
label.addText("Table/Row[3]/Cell[1..2]/Table/Row[1]/Cell[1]");
label.endCell();
label.endRow();
label.endTable();
label.endCell();
label.endRow();
label.endTable();
final GvNodeAttributes natts = new GvNodeAttributes();
natts.setFillColor(GvX11Colors.ALICEBLUE);
natts.setStyle(GvNodeStyle.FILLED);
natts.setShape(GvNodeShape.BOX);
natts.setPenWidth(1.0);
natts.setMargin(1.0);
natts.setLabel(label.toString());
writer.addNode("n1", natts);
}
writer.endGraph();
writer.addComment("End of graph");
writer.flush();
writer.close();
final GvToAny.MainArgs margs = new GvToAny.MainArgs();
margs.setEnabled(GvToAny.MainArgs.Feature.VERBOSE, true);
margs.input = new File(output);
margs.outputDir = new File(odir);
margs.engine = GvEngine.DOT;
margs.formats.add(GvFormat.PDF);
GvToAny.execute(margs);
System.out.println("Done");
} catch (final IOException e) {
e.printStackTrace();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy