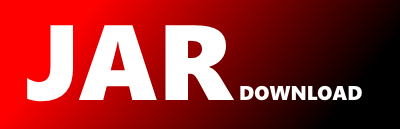
cdc.gv.demo.GvNodesDemo Maven / Gradle / Ivy
package cdc.gv.demo;
import java.io.File;
import java.io.IOException;
import cdc.gv.GvWriter;
import cdc.gv.atts.GvClusterAttributes;
import cdc.gv.atts.GvEdgeAttributes;
import cdc.gv.atts.GvEdgeStyle;
import cdc.gv.atts.GvGraphAttributes;
import cdc.gv.atts.GvLabelLoc;
import cdc.gv.atts.GvNodeAttributes;
import cdc.gv.atts.GvNodeShape;
import cdc.gv.atts.GvNodeStyle;
import cdc.gv.atts.GvRankDir;
import cdc.gv.colors.GvX11Colors;
import cdc.gv.tools.GvEngine;
import cdc.gv.tools.GvFormat;
import cdc.gv.tools.GvToAny;
import cdc.util.files.Files;
public final class GvNodesDemo {
private GvNodesDemo() {
}
private static String getId(String prefix,
int index) {
return prefix + index;
}
public static void main(String[] args) throws IOException {
final String odir = "target";
final String output = odir + "/" + GvNodesDemo.class.getSimpleName() + ".gv";
Files.mkdir(odir);
final GvWriter writer = new GvWriter(output);
try {
writer.addComment("Beginning of graph");
final GvGraphAttributes gatts = new GvGraphAttributes();
gatts.setRatio(1.0).setMaximumSize(10.0, 10.0).setRankDir(GvRankDir.LR).setNodeSep(0.0);
writer.beginGraph("test-nodes", true, gatts);
writer.println();
{
final String prefix = "shape";
final GvClusterAttributes sgatts = new GvClusterAttributes();
sgatts.setLabel("Node Shapes").setFontSize(60.0);
writer.beginCluster("shapes", sgatts);
final int width = (int) Math.ceil(Math.sqrt(GvNodeShape.values().length));
int index = 0;
for (final GvNodeShape shape : GvNodeShape.values()) {
final int pos = index % width;
index++;
final GvClusterAttributes catts = new GvClusterAttributes();
catts.setLabel(shape.name()).setLabelLoc(GvLabelLoc.BOTTOM).setMargin(0.2).setFontSize(12.0);
writer.beginCluster(getId(prefix, index), catts);
writer.addNode(getId(prefix, index),
new GvNodeAttributes()
.setShape(shape)
.setStyle(GvNodeStyle.FILLED)
.setLabel("")
.setFillColor(GvX11Colors.GRAY));
writer.endCluster();
if (pos > 0) {
final GvEdgeAttributes eatts = new GvEdgeAttributes();
eatts.setStyle(GvEdgeStyle.INVIS);
writer.addEdge(getId(prefix, index - 1), getId(prefix, index), eatts);
}
}
writer.endCluster();
}
{
final String prefix = "style";
final GvClusterAttributes sgatts = new GvClusterAttributes();
sgatts.setLabel("Node Styles").setFontSize(60.0);
writer.beginCluster("styles", sgatts);
int index = 0;
for (final GvNodeStyle style : GvNodeStyle.values()) {
index++;
final GvClusterAttributes catts = new GvClusterAttributes();
catts.setLabel(style.name()).setLabelLoc(GvLabelLoc.BOTTOM).setMargin(0.2).setFontSize(12.0);
writer.beginCluster(getId(prefix, index), catts);
{
final GvNodeAttributes natts = new GvNodeAttributes()
.setShape(GvNodeShape.OVAL)
.setStyle(style)
.setLabel("")
.setColor(GvX11Colors.BLACK);
if (style.supportsColorList()) {
natts.setFillColor(GvX11Colors.YELLOW, GvX11Colors.BLUE, GvX11Colors.RED);
} else {
natts.setFillColor(GvX11Colors.GRAY);
}
writer.addNode(getId(prefix + "Oval", index), natts);
}
{
final GvNodeAttributes natts = new GvNodeAttributes()
.setShape(GvNodeShape.BOX)
.setStyle(style)
.setLabel("")
.setColor(GvX11Colors.BLACK);
if (style.supportsColorList()) {
natts.setFillColor(GvX11Colors.YELLOW, GvX11Colors.BLUE, GvX11Colors.RED);
} else {
natts.setFillColor(GvX11Colors.GRAY);
}
writer.addNode(getId(prefix + "Box", index), natts);
}
writer.endCluster();
}
writer.endCluster();
}
writer.endGraph();
writer.addComment("End of graph");
writer.flush();
writer.close();
final GvToAny.MainArgs margs = new GvToAny.MainArgs();
margs.setEnabled(GvToAny.MainArgs.Feature.VERBOSE, true);
margs.input = new File(output);
margs.outputDir = new File(odir);
margs.engine = GvEngine.DOT;
margs.formats.add(GvFormat.PDF);
GvToAny.execute(margs);
System.out.println("Done");
} catch (final IOException e) {
e.printStackTrace();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy