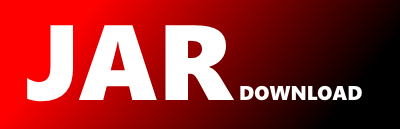
cdc.gv.demo.GvWriterDemo Maven / Gradle / Ivy
package cdc.gv.demo;
import java.io.File;
import java.io.IOException;
import cdc.gv.GvWriter;
import cdc.gv.atts.GvArrowType;
import cdc.gv.atts.GvDirType;
import cdc.gv.atts.GvEdgeAttributes;
import cdc.gv.atts.GvEdgeStyle;
import cdc.gv.atts.GvGraphAttributes;
import cdc.gv.atts.GvNodeAttributes;
import cdc.gv.atts.GvNodeShape;
import cdc.gv.atts.GvNodeStyle;
import cdc.gv.colors.GvColor;
import cdc.gv.colors.GvX11Colors;
import cdc.gv.labels.GvCellAttributes;
import cdc.gv.labels.GvHtmlLabel;
import cdc.gv.labels.GvTableAttributes;
import cdc.gv.labels.GvTextModifier;
import cdc.gv.tools.GvEngine;
import cdc.gv.tools.GvFormat;
import cdc.gv.tools.GvToAny;
import cdc.util.files.Files;
public final class GvWriterDemo {
private GvWriterDemo() {
}
private static String toLabel(String text) {
final GvHtmlLabel label = new GvHtmlLabel();
final GvTableAttributes tatts = new GvTableAttributes();
tatts.setBgColor(new GvColor(255, 0, 0));
tatts.setColor(new GvColor(0, 255, 0));
tatts.setBorder(4);
tatts.setCellBorder(6);
tatts.setCellPadding(6);
label.beginTable(tatts);
label.beginRow();
label.addCellImage("FALSE", "src/test/resources/java-class.png");
final GvCellAttributes catts = new GvCellAttributes();
catts.setBgColor(new GvColor(0, 255, 255));
label.beginCell(catts);
label.beginTextModifier(GvTextModifier.BOLD);
label.beginFont("#FF00FF", null, -1);
label.beginTextModifier(GvTextModifier.ITALIC);
label.beginTextModifier(GvTextModifier.UNDERLINE);
label.addText(text);
label.endTextModifier();
label.endTextModifier();
label.endFont();
label.endTextModifier();
label.endCell();
label.endRow();
label.endTable();
// System.err.println(label.toString());
// return text;
return label.toString();
}
public static void main(String[] args) throws IOException {
// final GvColor c1 = new GvColor(255, 128, 0);
// System.out.println(c1.encode());
// final GvColor c2 = new GvColor(1.0, 0.5, 0.0);
// System.out.println(c2.encode());
final String ouputDir = "target";
Files.mkdir(ouputDir);
final String gv = ouputDir + "/" + GvWriterDemo.class.getSimpleName() + ".gv";
final GvWriter writer = new GvWriter(gv);
try {
writer.addComment("Beginning of graph");
writer.beginGraph("test", true, new GvGraphAttributes().setRatio(10.0).setMaximumSize(10.0, 10.0));
writer.println();
writer.addComment("Nodes");
writer.addNode("n1",
new GvNodeAttributes()
.setShape(GvNodeShape.BOX)
.setLabel(toLabel("Node 1"))
.setStyle(GvNodeStyle.FILLED, GvNodeStyle.BOLD, GvNodeStyle.DASHED)
.setFillColor(GvX11Colors.ALICEBLUE));
writer.addNode("n2",
new GvNodeAttributes()
.setLabel(toLabel("Node 2"))
.setStyle(GvNodeStyle.FILLED)
.setFillColor(GvX11Colors.ANTIQUEWHITE));
writer.println();
writer.addComment("Edges");
writer.addEdge("n1",
"n2",
new GvEdgeAttributes()
.setLabel("Edge 1 2")
.setStyle(GvEdgeStyle.SOLID)
.setDir(GvDirType.BOTH)
.setArrowSize(2.0)
.setArrowHead(GvArrowType.BOX)
.setArrowTail(GvArrowType.DOT));
writer.println();
writer.addComment("Subgraph");
writer.beginSubgraph("sg1", null);
writer.beginCluster("c1", null);
writer.endCluster();
writer.endSubgraph();
writer.endGraph();
writer.addComment("End of graph");
writer.flush();
writer.close();
final GvToAny.MainArgs margs = new GvToAny.MainArgs();
margs.setEnabled(GvToAny.MainArgs.Feature.VERBOSE, true);
margs.input = new File(gv);
margs.outputDir = new File(ouputDir);
margs.engine = GvEngine.DOT;
margs.formats.add(GvFormat.GIF);
margs.formats.add(GvFormat.PS);
margs.formats.add(GvFormat.PS2);
margs.formats.add(GvFormat.JPG);
margs.formats.add(GvFormat.PNG);
margs.formats.add(GvFormat.PDF);
margs.formats.add(GvFormat.SVG);
margs.formats.add(GvFormat.SVGZ);
GvToAny.execute(margs);
System.out.println("Done");
} catch (final IOException e) {
e.printStackTrace();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy