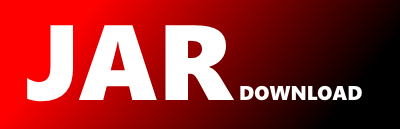
cdc.gv.demo.GvX11ColorsDemo Maven / Gradle / Ivy
package cdc.gv.demo;
import java.io.File;
import java.io.IOException;
import java.lang.reflect.Field;
import cdc.gv.GvWriter;
import cdc.gv.atts.GvEdgeAttributes;
import cdc.gv.atts.GvEdgeStyle;
import cdc.gv.atts.GvGraphAttributes;
import cdc.gv.atts.GvLabelLoc;
import cdc.gv.atts.GvNodeAttributes;
import cdc.gv.atts.GvNodeShape;
import cdc.gv.atts.GvNodeStyle;
import cdc.gv.colors.GvColor;
import cdc.gv.colors.GvX11Colors;
import cdc.gv.labels.GvHtmlLabel;
import cdc.gv.tools.GvEngine;
import cdc.gv.tools.GvFormat;
import cdc.gv.tools.GvToAny;
import cdc.util.files.Files;
/**
* Generate a GraphViz sample file with all X11 colors.
*
* @author Damien Carbonne
*
*/
public final class GvX11ColorsDemo {
private static String getId(Field field) {
return field.getName();
}
private GvX11ColorsDemo() {
}
public static void main(String[] args) {
final String odir = "target";
Files.mkdir(odir);
final String name = odir + "/" + GvX11ColorsDemo.class.getSimpleName() + ".gv";
try (final GvWriter writer = new GvWriter(name)) {
final GvGraphAttributes gatts = new GvGraphAttributes();
gatts.setLabel("X11 Colors");
gatts.setFontSize(100.0);
gatts.setFontName("Arial");
gatts.setLabelLoc(GvLabelLoc.TOP);
writer.beginGraph("test-x11-colors", true, gatts);
final int width = (int) Math.ceil(Math.sqrt(GvX11Colors.class.getDeclaredFields().length));
int index = 0;
Field previous = null;
for (final Field field : GvX11Colors.class.getDeclaredFields()) {
if (field.getType() == GvColor.class) {
final int pos = index % width;
// System.out.println(index + " " + pos);
// System.out.println(field.getName());
final GvColor color = (GvColor) field.get(GvX11Colors.class);
final GvHtmlLabel label = new GvHtmlLabel();
label.beginFont(GvX11Colors.BLACK, null, -1);
label.addText(field.getName());
label.endFont();
label.addLineBreak(null);
label.beginFont(GvX11Colors.WHITE, null, -1);
label.addText(field.getName());
label.endFont();
final GvNodeAttributes natts = new GvNodeAttributes();
natts.setColor(GvX11Colors.BLACK);
natts.setFillColor(color);
natts.setLabel(label.toString());
natts.setStyle(GvNodeStyle.FILLED);
natts.setShape(GvNodeShape.BOX);
writer.addNode(getId(field), natts);
if (pos > 0) {
final GvEdgeAttributes eatts = new GvEdgeAttributes();
eatts.setStyle(GvEdgeStyle.INVIS);
writer.addEdge(getId(previous), getId(field), eatts);
}
index++;
previous = field;
}
}
writer.endGraph();
writer.flush();
final GvToAny.MainArgs margs = new GvToAny.MainArgs();
margs.setEnabled(GvToAny.MainArgs.Feature.VERBOSE, true);
margs.input = new File(name);
margs.outputDir = new File(odir);
margs.engine = GvEngine.DOT;
margs.formats.add(GvFormat.PDF);
GvToAny.execute(margs);
System.out.println("Done");
} catch (final IOException | IllegalArgumentException | IllegalAccessException e) {
e.printStackTrace();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy