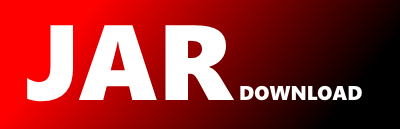
cdc.impex.ImpExCatalog Maven / Gradle / Ivy
The newest version!
package cdc.impex;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import cdc.impex.exports.SheetExporter;
import cdc.impex.exports.WorkbookExporter;
import cdc.impex.imports.SheetImporter;
import cdc.impex.imports.WorkbookImporter;
import cdc.impex.templates.SheetTemplate;
import cdc.util.lang.Checks;
import cdc.util.lang.NotFoundException;
/**
* Catalog of {@link SheetTemplate}s and {@link SheetImporter}s.
*
* @author Damien Carbonne
*/
public class ImpExCatalog {
private static final String TEMPLATE = "template";
private static final String TEMPLATES = "templates";
private static final String TEMPLATE_NAME = "templateName";
private static final String TEMPLATE_NAMES = "templateNames";
private static final String SHEET_EXPORTER = "sheetExporter";
private static final String SHEET_IMPORTER = "sheetImporter";
/**
* Map of (domain name, Set).
*/
private final Map> domainNameToTemplates = new HashMap<>();
/**
* Map of (template name, template).
*/
private final Map templateNameToTemplate = new HashMap<>();
/**
* Map of (template name, sheet importer).
*/
private final Map templateNameToSheetImporter = new HashMap<>();
/**
* Map of (template name, sheet exporter).
*/
private final Map templateNameToSheetExporter = new HashMap<>();
public ImpExCatalog() {
super();
}
/**
* Registers a SheetTemplate.
*
* @param template The sheet template.
* @return This catalog.
* @throws IllegalArgumentException When {@code template} is {@code null},
* or another template with that name is already registered.
*/
public ImpExCatalog register(SheetTemplate template) {
Checks.isNotNull(template, TEMPLATE);
Checks.doesNotContainKey(templateNameToTemplate, template.getName(), TEMPLATES);
templateNameToTemplate.put(template.getName(), template);
final Set set = domainNameToTemplates.computeIfAbsent(template.getDomain(), k -> new HashSet<>());
set.add(template);
return this;
}
/**
* Registers a collection of SheetTemplates.
*
* @param templates The sheet templates.
* @return This catalog.
* @throws IllegalArgumentException When {@code templates} is {@code null},
* or another template with same name is already registered.
*/
public ImpExCatalog register(Collection templates) {
for (final SheetTemplate template : templates) {
register(template);
}
return this;
}
/**
* Registers an array of SheetTemplates.
*
* @param templates The sheet templates.
* @return This catalog.
* @throws IllegalArgumentException When {@code templates} is {@code null},
* or another template with same name is already registered.
*/
public ImpExCatalog register(SheetTemplate... templates) {
return register(Arrays.asList(templates));
}
/**
* Registers a SheetImporter and associate it to a template name.
*
* @param templateName The template name.
* @param sheetImporter The sheet importer.
* @return This catalog.
* @throws IllegalArgumentException When {@code templateName} or {@code sheetImporter} is {@code null},
* or that would create a duplicate or no corresponding template is found.
*/
public ImpExCatalog register(String templateName,
SheetImporter sheetImporter) {
Checks.isNotNull(templateName, TEMPLATE_NAME);
Checks.isNotNull(sheetImporter, SHEET_IMPORTER);
Checks.containKey(templateNameToTemplate, templateName, TEMPLATES);
Checks.doesNotContainKey(templateNameToSheetImporter, templateName, "sheetImporters");
templateNameToSheetImporter.put(templateName, sheetImporter);
return this;
}
/**
* Registers a SheetTemplate (if not already done) and associate it to a SheetImporter.
*
* @param template The sheet template.
* @param sheetImporter The sheet importer.
* @return This catalog.
* @throws IllegalArgumentException When {@code template} or {@code sheetImporter} is {@code null},
* or that would create a duplicate.
*/
public ImpExCatalog register(SheetTemplate template,
SheetImporter sheetImporter) {
Checks.isNotNull(template, TEMPLATE);
Checks.isNotNull(sheetImporter, SHEET_IMPORTER);
if (!templateNameToTemplate.containsKey(template.getName())) {
register(template);
}
return register(template.getName(), sheetImporter);
}
/**
* Registers a SheetExporter and associate it to a template name.
*
* @param templateName The template name.
* @param sheetExporter The sheet exporter.
* @return This catalog.
* @throws IllegalArgumentException When {@code templateName} or {@code sheetExporter} is {@code null},
* or that would create a duplicate or no corresponding template is found.
*/
public ImpExCatalog register(String templateName,
SheetExporter sheetExporter) {
Checks.isNotNull(templateName, TEMPLATE_NAME);
Checks.isNotNull(sheetExporter, SHEET_EXPORTER);
Checks.containKey(templateNameToTemplate, templateName, TEMPLATES);
Checks.doesNotContainKey(templateNameToSheetExporter, templateName, SHEET_EXPORTER);
templateNameToSheetExporter.put(templateName, sheetExporter);
return this;
}
/**
* Registers a SheetTemplate (if not already done) and associate it to a SheetExporter.
*
* @param template The sheet template.
* @param sheetExporter The sheet exporter.
* @return This catalog.
* @throws IllegalArgumentException When {@code template} or {@code sheetExporter} is {@code null},
* or that would create a duplicate.
*/
public ImpExCatalog register(SheetTemplate template,
SheetExporter sheetExporter) {
Checks.isNotNull(template, TEMPLATE);
Checks.isNotNull(sheetExporter, SHEET_EXPORTER);
if (!templateNameToTemplate.containsKey(template.getName())) {
register(template);
}
return register(template.getName(), sheetExporter);
}
/**
* @return A set of domain names for which some sheet templates are registered.
*/
public Set getDomainNames() {
return domainNameToTemplates.keySet();
}
/**
* Returns a set of sheet templates associated to a domain.
*
* @param domain The domain.
* @return A set of sheet templates associated to {@code domain}.
*/
public Set getDomainTemplatesAsSet(String domain) {
return domainNameToTemplates.getOrDefault(domain, Collections.emptySet());
}
/**
* Returns a list of sheet templates associated to a domain.
*
* @param domain The domain.
* @return A list of sheet templates associated to {@code domain}.
*/
public List getDomainTemplatesAsList(String domain) {
return new ArrayList<>(getDomainTemplatesAsSet(domain));
}
/**
* @return A set of names of registered sheet templates.
*/
public Set getTemplateNames() {
return templateNameToTemplate.keySet();
}
/**
* @param templateName The template name.
* @return The registered sheet template named {@code name} or {@code null}.
*/
public SheetTemplate getTemplateOrNull(String templateName) {
return templateNameToTemplate.get(templateName);
}
/**
* @param templateName The template name.
* @return The registered sheet template named {@code name}.
* @throws NotFoundException When no sheet template named {@code templateName} is registered.
*/
public SheetTemplate getTemplate(String templateName) {
final SheetTemplate template = templateNameToTemplate.get(templateName);
if (template == null) {
throw new NotFoundException("No template named '" + templateName + "' found");
} else {
return template;
}
}
/**
* @param templateNames The template names.
* @return A set if templates from {@code templateNames}.
* @throws IllegalArgumentException When {@code templateNames} is {@code null}.
*/
public Set getTemplatesAsSet(Collection templateNames) {
Checks.isNotNull(templateNames, TEMPLATE_NAMES);
final Set set = new HashSet<>();
for (final String name : templateNames) {
set.add(getTemplate(name));
}
return set;
}
public List getTemplatesAsList(Collection templateNames) {
Checks.isNotNull(templateNames, TEMPLATE_NAMES);
final List list = new ArrayList<>();
for (final String name : templateNames) {
list.add(getTemplate(name));
}
return list;
}
/**
* @param templateNames The template names.
* @return A set if templates from {@code templateNames}.
* @throws IllegalArgumentException When {@code templateNames} is {@code null}.
*/
public Set getTemplatesAsSet(String... templateNames) {
return getTemplatesAsSet(Arrays.asList(templateNames));
}
public List getTemplatesAsList(String... templateNames) {
return getTemplatesAsList(Arrays.asList(templateNames));
}
/**
* @return A set of sheet template names for which an sheet importer is registered.
*/
public Set getImportableTemplateNames() {
return templateNameToSheetImporter.keySet();
}
/**
* @return A set of sheet template names for which an sheet exporter is registered.
*/
public Set getExtractableTemplateNames() {
return templateNameToSheetExporter.keySet();
}
/**
* @return A set of all registered templates.
*/
public Set getTemplates() {
final Set set = new HashSet<>();
set.addAll(templateNameToTemplate.values());
return set;
}
/**
* Returns sheet importer associated to a template name or {@code null}.
*
* @param templateName The template name.
* @return The sheet importer associated to {@code templateName} or {@code null}.
*/
public SheetImporter getSheetImporterOrNull(String templateName) {
return templateNameToSheetImporter.get(templateName);
}
/**
* Return The sheet importer associated to template name.
*
* @param templateName The template name.
* @return The sheet importer associated to {@code templateName}.
* @throws NotFoundException When no sheet importer is associated to {@code templateName}.
*/
public SheetImporter getSheetImporter(String templateName) {
final SheetImporter sheetImporter = templateNameToSheetImporter.get(templateName);
if (sheetImporter == null) {
throw new NotFoundException("No sheet importer associated to template '" + templateName + "' found");
} else {
return sheetImporter;
}
}
/**
* Creates a delegating sheet importer for a collection of template names.
*
* @param def The default sheet importer.
* @param templateNames The template names.
* @return A newly created sheet importer.
*/
public WorkbookImporter createWorkbookImporterFor(WorkbookImporter def,
Collection templateNames) {
final Map map = new HashMap<>();
for (final String templateName : templateNames) {
map.put(templateName, getSheetImporter(templateName));
}
return WorkbookImporter.fromDelegates(def, map);
}
public WorkbookImporter createWorkbookImporterFor(Collection templateNames) {
return createWorkbookImporterFor(WorkbookImporter.QUIET_VOID, templateNames);
}
/**
* Creates a delegating sheet importer for an array of template names.
*
* @param def The default sheet importer.
* @param templateNames The template names.
* @return A newly created sheet importer.
*/
public WorkbookImporter createWorkbookImporterFor(WorkbookImporter def,
String... templateNames) {
return createWorkbookImporterFor(def, Arrays.asList(templateNames));
}
public WorkbookImporter createWorkbookImporterFor(String... templateNames) {
return createWorkbookImporterFor(WorkbookImporter.QUIET_VOID, templateNames);
}
/**
* Returns sheet exporter associated to a template name or {@code null}.
*
* @param templateName The template name.
* @return The sheet exporter associated to {@code templateName} or {@code null}.
*/
public SheetExporter getSheetExporterOrNull(String templateName) {
return templateNameToSheetExporter.get(templateName);
}
/**
* Return The sheet exporter associated to template name.
*
* @param templateName The template name.
* @return The sheet exporter associated to {@code templateName}.
* @throws NotFoundException When no sheet exporter is associated to {@code templateName}.
*/
public SheetExporter getSheetExporter(String templateName) {
final SheetExporter sheetExporter = templateNameToSheetExporter.get(templateName);
if (sheetExporter == null) {
throw new NotFoundException("No sheet exporter associated to template '" + templateName + "' found");
} else {
return sheetExporter;
}
}
/**
* Creates a delegating sheet exporter for a collection of template names.
*
* @param def The default sheet exporter.
* @param templateNames The template names.
* @return A newly created sheet exporter.
*/
public WorkbookExporter createWorkbookExporterFor(WorkbookExporter def,
Collection templateNames) {
final Map map = new HashMap<>();
for (final String templateName : templateNames) {
map.put(templateName, getSheetExporter(templateName));
}
return WorkbookExporter.fromDelegates(def, map);
}
public WorkbookExporter createWorkbookExporterFor(Collection templateNames) {
return createWorkbookExporterFor(WorkbookExporter.QUIET_VOID, templateNames);
}
/**
* Creates a delegating sheet exporter for an array of template names.
*
* @param def The default sheet exporter.
* @param templateNames The template names.
* @return A newly created sheet exporter.
*/
public WorkbookExporter createWorkbookExporterFor(WorkbookExporter def,
String... templateNames) {
return createWorkbookExporterFor(def, Arrays.asList(templateNames));
}
public WorkbookExporter createWorkbookExporterFor(String... templateNames) {
return createWorkbookExporterFor(WorkbookExporter.QUIET_VOID, templateNames);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy