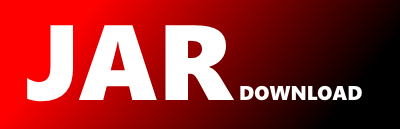
cdc.impex.ImpExFactory Maven / Gradle / Ivy
package cdc.impex;
import java.io.File;
import cdc.impex.exports.ActiveExporter;
import cdc.impex.exports.Exporter;
import cdc.impex.exports.StreamExporter;
import cdc.impex.imports.ImportAnalyzer;
import cdc.impex.imports.Importer;
import cdc.impex.templates.TemplateGenerator;
import cdc.issues.Issue;
import cdc.issues.IssuesHandler;
import cdc.util.lang.Checks;
import cdc.util.lang.FailureReaction;
import cdc.util.lang.Introspection;
/**
* Factory of {@link Importer}s, {@link StreamExporter}s and {@link TemplateGenerator}s.
*
* Note:At the moment, CSV, XLS, XSLX, XLSM and ODS workbooks are supported.
* In the future, some other formats (such as XML or JSON) may/will be supported.
*
* @author Damien Carbonne
*/
public class ImpExFactory {
private final ImpExFactoryFeatures features;
private static final String FORMAT = "format";
private static final String FILE = "file";
public ImpExFactory(ImpExFactoryFeatures features) {
this.features = features;
}
public ImpExFactoryFeatures getFeatures() {
return features;
}
/**
* @param file The file
* @return {@code true} if {@code file} can be imported.
* WARNING: {@code file} extension is checked.
*/
public boolean canImportFrom(File file) {
if (file == null) {
return false;
} else {
final ImpExFormat format = ImpExFormat.from(file);
return format != null && format.isImportFormat();
}
}
/**
* Creates an Importer for a file.
*
* @param file The file.
* @return An Importer that can import from {@code file} or any compliant file.
* @throws IllegalArgumentException When {@code file} is {@code null} or extension is not recognized.
*/
public Importer createImporter(File file) {
Checks.isNotNull(file, "file");
final ImpExFormat format = ImpExFormat.from(file);
if (format != null) {
switch (format) {
case CSV, ODS, XLS, XLSM, XLSX:
return createImporter("cdc.impex.core.workbooks.WorkbookImporterImpl");
case JSON:
return createImporter("cdc.impex.core.json.JsonImporterImpl");
default:
break;
}
}
throw new IllegalArgumentException("Can not create an Importer for " + file);
}
private Importer createImporter(String className) {
final Class extends Importer> cls =
Introspection.uncheckedCast(Introspection.getClass(className,
Importer.class,
FailureReaction.FAIL));
final Class>[] parameterTypes = { ImpExFactory.class };
return Introspection.newInstance(cls, parameterTypes, FailureReaction.FAIL, this);
}
/**
* @param file The file
* @return {@code true} if data cab be exported to {@code file}.
* WARNING: {@code file} extension is checked.
*/
public boolean canExportTo(File file) {
if (file == null) {
return false;
} else {
final ImpExFormat format = ImpExFormat.from(file);
return format != null && format.isExportFormat();
}
}
/**
* Creates a {@link StreamExporter} for a format.
*
* @param format The format.
* @param issuesHandler The issues handler.
* @return A {@link StreamExporter} compliant with {@code format}.
* @throws IllegalArgumentException When {@code format} or {@code issuesHandler} is {@code null}.
*/
public StreamExporter createStreamExporter(ImpExFormat format,
IssuesHandler issuesHandler) {
Checks.isNotNull(format, FORMAT);
Checks.isNotNull(issuesHandler, "issuesHandler");
switch (format) {
case CSV, ODS, XLS, XLSM, XLSX:
return createStreamExporter("cdc.impex.core.workbooks.WorkbookStreamExporter", issuesHandler);
case JSON:
return createStreamExporter("cdc.impex.core.json.JsonStreamExporter", issuesHandler);
case XML:
return createStreamExporter("cdc.impex.core.xml.XmlStreamExporter", issuesHandler);
default:
break;
}
throw new IllegalArgumentException("Can not create a " + StreamExporter.class.getSimpleName() + " for " + format);
}
/**
* Creates a {@link StreamExporter} compliant with a file extension.
*
* @param file The file.
* @param issuesHandler The issues handler.
* @return A {@link StreamExporter} that can export to {@code file} or any other compliant file.
* @throws IllegalArgumentException When {@code file} or {@code issuesHandler} is {@code null},
* or file extension is not recognized.
*/
public StreamExporter createStreamExporter(File file,
IssuesHandler issuesHandler) {
Checks.isNotNull(file, FILE);
Checks.isNotNull(issuesHandler, "issuesHandler");
final ImpExFormat format = ImpExFormat.from(file);
return createStreamExporter(format, issuesHandler);
}
private StreamExporter createStreamExporter(String className,
IssuesHandler issuesHandler) {
final Class extends StreamExporter> cls =
Introspection.uncheckedCast(Introspection.getClass(className,
StreamExporter.class,
FailureReaction.FAIL));
final Class>[] parameterTypes = { IssuesHandler.class, ImpExFactory.class };
return Introspection.newInstance(cls, parameterTypes, FailureReaction.FAIL, issuesHandler, this);
}
/**
* Creates an {link Exporter} implementation that is compliant with the format of a file.
*
* @param file The file.
* @return An {link Exporter} implementation compliant with format of {@code file}.
* @throws IllegalArgumentException When {@code file} is {@code null}
* or its format is not supported.
*/
public Exporter createExporter(File file) {
Checks.isNotNull(file, FILE);
final ImpExFormat format = ImpExFormat.from(file);
if (format == null) {
throw new IllegalArgumentException("Can not create an Exporter for " + file);
}
return createExporter(format);
}
/**
* Creates an {link Exporter} implementation that is compliant with a format.
*
* @param format The format.
* @return An {link Exporter} implementation compliant with {@code format}.
* @throws IllegalArgumentException When {@code format} is {@code null}.
*/
public Exporter createExporter(ImpExFormat format) {
Checks.isNotNull(format, FORMAT);
return createExporter("cdc.impex.core.ExporterImpl");
}
private Exporter createExporter(String className) {
final Class extends Exporter> cls =
Introspection.uncheckedCast(Introspection.getClass(className,
Exporter.class,
FailureReaction.FAIL));
final Class>[] parameterTypes = { ImpExFactory.class };
return Introspection.newInstance(cls, parameterTypes, FailureReaction.FAIL, this);
}
/**
* Creates an {link ActiveExporter} implementation that is compliant with the format of a file.
*
* @param file The file.
* @return An {link ActiveExporter} implementation compliant with format of {@code file}.
* @throws IllegalArgumentException When {@code file} is {@code null}
* or its format is not supported.
*/
public ActiveExporter createActiveExporter(File file) {
Checks.isNotNull(file, FILE);
final ImpExFormat format = ImpExFormat.from(file);
if (format == null) {
throw new IllegalArgumentException("Can not create an Exporter for " + file);
}
return createActiveExporter(format);
}
/**
* Creates an {link ActiveExporter} implementation that is compliant with a format.
*
* @param format The format.
* @return An {link ActiveExporter} implementation compliant with {@code format}.
* @throws IllegalArgumentException When {@code format} is {@code null}.
*/
public ActiveExporter createActiveExporter(ImpExFormat format) {
Checks.isNotNull(format, FORMAT);
return createActiveExporter("cdc.impex.core.ActiveExporterImpl");
}
private ActiveExporter createActiveExporter(String className) {
final Class extends ActiveExporter> cls =
Introspection.uncheckedCast(Introspection.getClass(className,
ActiveExporter.class,
FailureReaction.FAIL));
final Class>[] parameterTypes = { ImpExFactory.class };
return Introspection.newInstance(cls, parameterTypes, FailureReaction.FAIL, this);
}
/**
* Creates a TemplateGenarator for a file.
*
* @param file The file.
* @return A TemplateGenrator that matches {@code file}. It can be used with any compliant file.
* @throws IllegalArgumentException When {@code file} is {@code null} or extension is not recognized.
*/
public TemplateGenerator createTemplateGenerator(File file) {
Checks.isNotNull(file, "file");
final ImpExFormat format = ImpExFormat.from(file);
if (format != null) {
switch (format) {
case CSV, ODS, XLS, XLSM, XLSX:
return createTemplateGenerator("cdc.impex.core.workbooks.WorkbookTemplateGenerator");
case JSON:
return createTemplateGenerator("cdc.impex.core.json.JsonTemplateGenerator");
default:
break;
}
}
throw new IllegalArgumentException("Can not create a TemplateGenerator for " + file);
}
private TemplateGenerator createTemplateGenerator(String className) {
final Class extends TemplateGenerator> cls =
Introspection.uncheckedCast(Introspection.getClass(className,
TemplateGenerator.class,
FailureReaction.FAIL));
final Class>[] parameterTypes = { ImpExFactory.class };
return Introspection.newInstance(cls, parameterTypes, FailureReaction.FAIL, this);
}
public ImportAnalyzer createImportAnalyzer(File file) {
Checks.isNotNull(file, FILE);
final ImpExFormat format = ImpExFormat.from(file);
if (format != null) {
switch (format) {
case CSV, ODS, XLS, XLSM, XLSX, JSON:
return createImportAnalyzer("cdc.impex.core.ImportAnalyzerImpl");
default:
break;
}
}
throw new IllegalArgumentException("Can not create an ImportAnalyzer for " + file);
}
private ImportAnalyzer createImportAnalyzer(String className) {
final Class extends ImportAnalyzer> cls =
Introspection.uncheckedCast(Introspection.getClass(className,
ImportAnalyzer.class,
FailureReaction.FAIL));
final Class>[] parameterTypes = { ImpExFactory.class };
return Introspection.newInstance(cls, parameterTypes, FailureReaction.FAIL, this);
}
}