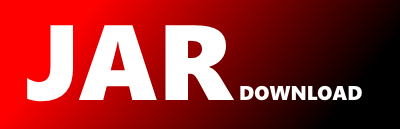
cdc.impex.ImpExFactoryFeatures Maven / Gradle / Ivy
package cdc.impex;
import java.util.EnumSet;
import java.util.Optional;
import java.util.Set;
import cdc.impex.templates.ImportAction;
import cdc.office.ss.WorkbookWriterFeatures;
import cdc.util.lang.Checks;
public final class ImpExFactoryFeatures {
public static final ImpExFactoryFeatures BEST =
builder().workbookWriterFeatures(WorkbookWriterFeatures.STANDARD_BEST)
.hint(Hint.ADD_HEADER_COMMENTS)
.hint(Hint.ADD_DATA_COMMENTS)
.hint(Hint.ADD_CONTENT_VALIDATION)
.hint(Hint.ADD_README)
.hint(Hint.PRETTY_PRINT)
.build();
public static final ImpExFactoryFeatures BEST_NO_ACTION =
builder().workbookWriterFeatures(WorkbookWriterFeatures.STANDARD_BEST)
.hint(Hint.ADD_HEADER_COMMENTS)
.hint(Hint.ADD_DATA_COMMENTS)
.hint(Hint.ADD_CONTENT_VALIDATION)
.hint(Hint.ADD_README)
.hint(Hint.PRETTY_PRINT)
.hint(Hint.SKIP_ACTION_COLUMN)
.build();
public static final ImpExFactoryFeatures FASTEST =
builder().workbookWriterFeatures(WorkbookWriterFeatures.STANDARD_FAST)
.hint(Hint.PRETTY_PRINT)
.hint(Hint.POI_STREAMING)
.build();
public static final ImpExFactoryFeatures FASTEST_NO_ACTION =
builder().workbookWriterFeatures(WorkbookWriterFeatures.STANDARD_FAST)
.hint(Hint.PRETTY_PRINT)
.hint(Hint.POI_STREAMING)
.hint(Hint.SKIP_ACTION_COLUMN)
.build();
private final WorkbookWriterFeatures workbookWriterFeatures;
private final Set hints = EnumSet.noneOf(Hint.class);
private final String password;
private final Optional defaultAction;
private ImpExFactoryFeatures(Builder builder) {
this.workbookWriterFeatures = Checks.isNotNull(builder.workbookWriterFeatures, "workbookWriterFeatures");
this.hints.addAll(builder.hints);
this.password = builder.password;
this.defaultAction = Optional.ofNullable(builder.defaultAction);
}
public enum Hint {
/**
* If enabled, adds comments to headers if possible.
*
* Specific to Workbooks.
*/
ADD_HEADER_COMMENTS,
/**
* If enabled, adds data comments if possible and meaningful.
*/
ADD_DATA_COMMENTS,
/**
* If enabled, adds content validation if possible.
*
* Specific to Workbooks.
*/
ADD_CONTENT_VALIDATION,
/**
* If enabled, adds README sections.
*/
ADD_README,
/**
* If enabled, do not print action column.
*/
SKIP_ACTION_COLUMN,
/**
* If enabled, pretty prints result.
*/
PRETTY_PRINT,
/**
* If enabled, uses Fast ODS.
*
* Specific to Workbooks.
* WARNING: This is still experimental and incomplete. Do not use.
*/
ODS_FAST,
/**
* If enabled, uses ODF Toolkit simple API.
*
* Specific to Workbooks.
* WARNING: This is very slow, but seems to work.
*/
ODS_SIMPLE,
/**
* If enabled, uses POI Streaming API.
*
* Specific to Workbooks.
* This requires less memory, but is not compliant with certain features.
*/
POI_STREAMING,
/**
* If enabled and Action column is missing, continue import processing.
*
* Specific to Workbooks.
*/
IGNORE_MISSING_ACTION_COLUMN
}
/**
* @return The Workbook Writer features.
*/
public WorkbookWriterFeatures getWorkbookWriterFeatures() {
return workbookWriterFeatures;
}
/**
* @param hint The hint.
* @return {@code true} if {@code hint} is enabled.
*/
public boolean isEnabled(Hint hint) {
return hints.contains(hint);
}
/**
* @return The password (possibly {@code null}).
*/
public String getPassword() {
return password;
}
/**
* @return The default action to use.
* When defined, it overrides the default action defined in sheet templates.
*/
public Optional getDefaultAction() {
return defaultAction;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private WorkbookWriterFeatures workbookWriterFeatures = WorkbookWriterFeatures.STANDARD_FAST;
private final Set hints = EnumSet.noneOf(Hint.class);
private String password = null;
private ImportAction defaultAction = null;
protected Builder() {
super();
}
public Builder workbookWriterFeatures(WorkbookWriterFeatures features) {
this.workbookWriterFeatures = features;
return this;
}
public Builder hint(Hint hint) {
hints.add(hint);
return this;
}
public Builder password(String password) {
this.password = password;
return this;
}
public Builder defaultAction(ImportAction defaultAction) {
this.defaultAction = defaultAction;
return this;
}
public ImpExFactoryFeatures build() {
return new ImpExFactoryFeatures(this);
}
}
}