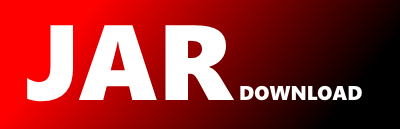
cdc.impex.exports.ActiveExporter Maven / Gradle / Ivy
package cdc.impex.exports;
import java.io.File;
import java.io.IOException;
import java.io.OutputStream;
import cdc.impex.ImpExFormat;
import cdc.impex.templates.SheetTemplate;
import cdc.impex.templates.SheetTemplateInstance;
import cdc.issues.Issue;
import cdc.issues.IssueSeverity;
import cdc.issues.IssuesHandler;
import cdc.util.events.ProgressController;
/**
* Interface dedicated to export and that adopts the active iterator approach.
*
* [Storage Device] → [Memory] → {@link ActiveExporter} → [File/Stream].
*
* The calling sequence must be:
* {@code beginExport (beginSheet (nextRow addRow)* endSheet)* endExport}
*
* @author Damien Carbonne
*/
public interface ActiveExporter {
/**
* Called once for a file.
*
* @param file The file.
* @param issuesHandler The issues handler.
* @param controller The controller
* @throws IOException When an IO error occurs.
*/
public void beginExport(File file,
IssuesHandler issuesHandler,
ProgressController controller) throws IOException;
/**
* Called once for an output stream.
*
* @param out The output stream.
* @param systemId The system id.
* @param format The output format.
* @param issuesHandler The issues handler.
* @param controller The controller
* @throws IOException When an IO error occurs.
*/
public void beginExport(OutputStream out,
String systemId,
ImpExFormat format,
IssuesHandler issuesHandler,
ProgressController controller) throws IOException;
/**
* Called once for each logical sheet.
*
* One logical sheet can generate several physical sheets, depending on the limits of the output format.
*
* WARNING: the version must be called when {@code template} contains pattern columns.
*
* @param templateInstance The template instance.
* @param numberOfRows The number of rows in the logical sheet.
* Pass -1 if that number is unknown.
* @throws IOException When an IO error occurs.
*/
public void beginSheet(SheetTemplateInstance templateInstance,
long numberOfRows) throws IOException;
/**
* Called once for each logical sheet.
*
* One logical sheet can generate several physical sheets, depending on the limits of the output format.
*
* WARNING: the version can be called when {@code template} does not contain any pattern column.
*
* @param template The template.
* @param numberOfRows The number of rows in the logical sheet.
* Pass -1 if that number is unknown.
* @throws IOException When an IO error occurs.
*/
public void beginSheet(SheetTemplate template,
long numberOfRows) throws IOException;
/**
* Called once for each row.
*
* @return The row to fill, cleared and prepared.
* @throws IOException When an IO error occurs.
*/
public ExportRow nextRow() throws IOException;
/**
* Called once for each row, once it has been filled.
*
* @throws IOException When an IO error occurs.
*/
public void addRow() throws IOException;
/**
* Called once at the end of each logical sheet.
*
* @throws IOException When an IO error occurs.
*/
public void endSheet() throws IOException;
/**
* Called once at the end of export.
*
* @throws IOException When an IO error occurs.
*/
public void endExport() throws IOException;
/**
* @return The used issues handler.
*/
public IssuesHandler getIssuesHandler();
/**
* Creates a new issue.
*
* @param type The issue type.
* @param severity The issue severity.
* @param description The issue description.
*/
public void issue(ExportIssueType type,
IssueSeverity severity,
String description);
/**
* Creates a new issue whose severity is deduced from its type.
*
* @param type The issue type.
* @param description The issue description.
*/
public default void issue(ExportIssueType type,
String description) {
issue(type, type.getSeverity(), description);
}
}