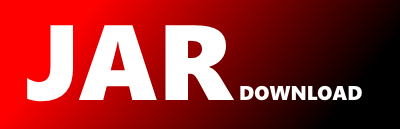
cdc.impex.exports.ExportRow Maven / Gradle / Ivy
package cdc.impex.exports;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.util.List;
import cdc.impex.templates.ColumnTemplate;
import cdc.impex.templates.SheetTemplate;
import cdc.impex.templates.SheetTemplateInstance;
import cdc.issues.Issue;
public interface ExportRow {
/**
* @return The associated template instance.
*/
public SheetTemplateInstance getTemplateInstance();
/**
* @return The associated template.
*/
public SheetTemplate getTemplate();
/**
* @return The name of the sheet for which row is generated.
*/
public String getSheetName();
/**
* Returns the 1-based number of this row.
*
* In a sheet, the first row is numbered 2 whether the used format has headers or not.
* A header row numbered 1 is always considered, even if it does not exist with the used format.
*
* @return The 1-based number of this row.
*/
public int getNumber();
/**
* Set the data associated to a column
*
* @param name The column name.
* @param data The data.
* @throws IllegalArgumentException When {@code name} or {@code data} is invalid.
*/
public void setData(String name,
Object data);
/**
* Set the data associated to a name column.
*
* WARNING: MUST be used with a name column.
*
* @param The column data type.
* @param column The column.
* @param data The data.
* @throws IllegalArgumentException When {@code name} or {@code data} is invalid.
*/
public void setData(ColumnTemplate column,
T data);
/**
* Set the data associated to a column.
*
* This is mainly intended for pattern columns, but can be used with any column, as long as
* {@code name} is compliant with {@code column}.
*
* @param The column data type.
* @param column The column.
* @param name The name, which must match {@code column}.
* @param data The data.
*/
public void setData(ColumnTemplate column,
String name,
T data);
/**
* @return The issues associated to this row.
*/
public List getIssues();
/**
* @param name The column name.
* @return {@code true} if a value is associated to {@code name}.
*/
public boolean containsKey(String name);
public String getValue(String name);
public boolean hasValidValue(String name);
public Boolean getValueAsBoolean(String name);
public Long getValueAsLong(String name);
public Double getValueAsDouble(String name);
public BigInteger getValueAsBigInteger(String name);
public BigDecimal getValueAsBigDecimal(String name);
public String getComment(String name);
}