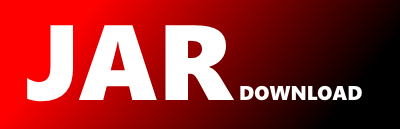
cdc.impex.exports.Exporter Maven / Gradle / Ivy
package cdc.impex.exports;
import java.io.File;
import java.io.IOException;
import java.io.OutputStream;
import java.util.List;
import java.util.function.Function;
import cdc.impex.ImpExCatalog;
import cdc.impex.ImpExFactory;
import cdc.impex.ImpExFactoryFeatures;
import cdc.impex.ImpExFormat;
import cdc.impex.templates.SheetTemplate;
import cdc.impex.templates.SheetTemplateInstance;
import cdc.issues.Issue;
import cdc.issues.IssuesHandler;
import cdc.util.events.ProgressController;
/**
* Interface implemented by classes that can export data to a file or stream.
*
* @author Damien Carbonne
*/
public interface Exporter {
/**
* Export data to a file.
*
* @param file The file to generate.
* @param templateInstances The list of {@link SheetTemplateInstance template instances} for which sheets must be generated.
* @param workbookExporter The workbook exporter to be used to extract data to export.
* @param issuesHandler The issues handler.
* @param controller The controller.
* @throws IOException When an IO error occurs.
* @throws IllegalArgumentException When any input parameter is {@code null}.
*/
public void exportData(File file,
List templateInstances,
WorkbookExporter workbookExporter,
IssuesHandler issuesHandler,
ProgressController controller) throws IOException;
/**
* Export data to an output stream using a format.
*
* @param out The output stream.
* @param systemId The system id.
* @param format The format.
* @param templateInstances The list of {@link SheetTemplateInstance template instances} for which sheets must be generated.
* @param workbookExporter The workbook exporter to be used to extract data to export.
* @param issuesHandler The issues handler.
* @param controller The controller.
* @throws IOException When an IO error occurs.
* @throws IllegalArgumentException When any input parameter (except {@code systemId}) is {@code null}.
*/
public void exportData(OutputStream out,
String systemId,
ImpExFormat format,
List templateInstances,
WorkbookExporter workbookExporter,
IssuesHandler issuesHandler,
ProgressController controller) throws IOException;
/**
* Exports data to a file using an {@link ImpExCatalog}.
*
* @param file The file to generate.
* @param templateNames The list of template names.
* @param catalog The catalog.
* @param instancier The function that converts each {@link SheetTemplate template} to a {@link SheetTemplateInstance
* template instance}.
* @param issuesHandler The issues handler.
* @param controller The controller.
* @param features The features.
* @throws IOException When an IO error occurs.
*/
public static void exportData(File file,
List templateNames,
ImpExCatalog catalog,
Function instancier,
IssuesHandler issuesHandler,
ProgressController controller,
ImpExFactoryFeatures features) throws IOException {
final ImpExFactory factory = new ImpExFactory(features);
final Exporter exporter = factory.createExporter(file);
exporter.exportData(file,
catalog.getTemplatesAsList(templateNames).stream().map(instancier::apply).toList(),
catalog.createWorkbookExporterFor(templateNames),
issuesHandler,
controller);
}
/**
* Exports data to a file using an {@link ImpExCatalog}.
*
* WARNING: this must be used when all template headers contain only names.
*
* @param file The file to generate.
* @param templateNames The list of template names.
* @param catalog The catalog.
* @param issuesHandler The issues handler.
* @param controller The controller.
* @param features The features.
* @throws IOException When an IO error occurs.
*/
public static void exportData(File file,
List templateNames,
ImpExCatalog catalog,
IssuesHandler issuesHandler,
ProgressController controller,
ImpExFactoryFeatures features) throws IOException {
exportData(file,
templateNames,
catalog,
SheetTemplateInstance::of,
issuesHandler,
controller,
features);
}
/**
* Exports data to a stream using an {@link ImpExCatalog}.
*
* @param out The output stream.
* @param systemId The system id.
* @param format The format.
* @param templateNames The list of template names.
* @param catalog The catalog.
* @param instancier The function that converts each {@link SheetTemplate template} to a {@link SheetTemplateInstance
* template instance}.
* @param issuesHandler The issues handler.
* @param controller The controller.
* @param features The features.
* @throws IOException When an IO error occurs.
*/
public static void exportData(OutputStream out,
String systemId,
ImpExFormat format,
List templateNames,
ImpExCatalog catalog,
Function instancier,
IssuesHandler issuesHandler,
ProgressController controller,
ImpExFactoryFeatures features) throws IOException {
final ImpExFactory factory = new ImpExFactory(features);
final Exporter exporter = factory.createExporter(format);
exporter.exportData(out,
systemId,
format,
catalog.getTemplatesAsList(templateNames).stream().map(instancier::apply).toList(),
catalog.createWorkbookExporterFor(templateNames),
issuesHandler,
controller);
}
/**
* Exports data to a stream using an {@link ImpExCatalog}.
*
* WARNING: this must be used when all template headers contain only names.
*
* @param out The output stream.
* @param systemId The system id.
* @param format The format.
* @param templateNames The list of template names.
* @param catalog The catalog.
* @param issuesHandler The issues handler.
* @param controller The controller.
* @param features The features.
* @throws IOException When an IO error occurs.
*/
public static void exportData(OutputStream out,
String systemId,
ImpExFormat format,
List templateNames,
ImpExCatalog catalog,
IssuesHandler issuesHandler,
ProgressController controller,
ImpExFactoryFeatures features) throws IOException {
exportData(out,
systemId,
format,
templateNames,
catalog,
SheetTemplateInstance::of,
issuesHandler,
controller,
features);
}
}