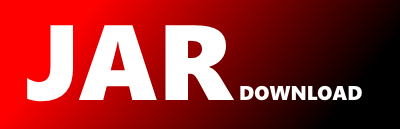
cdc.impex.exports.SheetExporter Maven / Gradle / Ivy
package cdc.impex.exports;
import cdc.impex.templates.SheetTemplate;
import cdc.impex.templates.SheetTemplateInstance;
import cdc.issues.Issue;
import cdc.issues.IssuesHandler;
/**
* Interface implemented by classes that can export data from storage device to memory.
*
* [Storage Device] → {@link SheetExporter} → [Memory] → {@link Exporter} → [File/Stream].
*
* A {@link SheetExporter} can export data corresponding to different (at least one) {@link SheetTemplate}s.
*
* @author Damien Carbonne
*/
public interface SheetExporter {
public static final SheetExporter QUIET_VOID = new CheckedSheetExporter();
public static final SheetExporter VERBOSE_VOID = new VerboseSheetExporter(QUIET_VOID);
/**
* Invoked to begin a sheet exports.
*
* @param templateInstance The sheet template instance associated to this extraction.
* @param issuesHandler The issues handler that should be used by the application to
* generate new issues.
*/
public default void beginSheetExport(SheetTemplateInstance templateInstance,
IssuesHandler issuesHandler) {
// Ignore
}
/**
* @return The remaining number of rows to extract in current row.
* It can be an approximation when {@code > 0}.
* WARNING: This must be 0 when there are no more rows to export.
* WARNING: This must be a negative number when the number is unknown.
*/
public int getNumberOfRemainingRows();
/**
* @return {@code true} if there are no more rows to extract in current sheet.
*/
public default boolean hasMore() {
return getNumberOfRemainingRows() != 0;
}
/**
* Invoked to export the current row in current sheet.
*
* @param row The row that will contain extracted data.
* @param issuesHandler The issues handler that should be used by the application to
* generate new issues.
*/
public void exportRow(ExportRow row,
IssuesHandler issuesHandler);
/**
* Invoked to end a sheet export.
*
* @param templateInstance The sheet template instance associated to this extraction.
* @param issuesHandler The issues handler that should be used by the application to
* generate new issues.
*/
public default void endSheetExport(SheetTemplateInstance templateInstance,
IssuesHandler issuesHandler) {
// Ignore
}
}