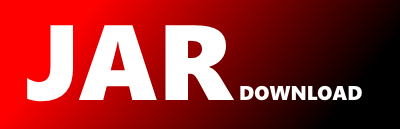
cdc.impex.exports.StreamExporter Maven / Gradle / Ivy
package cdc.impex.exports;
import java.io.Closeable;
import java.io.File;
import java.io.Flushable;
import java.io.IOException;
import java.io.OutputStream;
import java.nio.charset.Charset;
import java.util.List;
import cdc.impex.ImpExFactoryFeatures;
import cdc.impex.ImpExFormat;
import cdc.impex.templates.SheetTemplateInstance;
import cdc.office.ss.WorkbookWriterFeatures;
/**
* Interface implemented by classes that can be used to export data.
*
* This is a low level API that should be used like this:
*
* beginExport(file/stream);
* foreach (sheet):
* beginSheet(sheet.template);
* foreach (row):
* beginRow();
* foreach(column):
* setData(column, data);
* endRow();
* endSheet();
* endExport();
*
*
* @author Damien Carbonne
*/
public interface StreamExporter extends Flushable, Closeable {
/**
* Must be invoked at beginning of export to a {@link File}.
*
* The used {@link Charset} is the one defined in {@link WorkbookWriterFeatures}
* associated to the {@link ImpExFactoryFeatures}.
*
* @param file The target file.
* @throws IOException When a IO error occurs.
* @throws IllegalArgumentException When {@code file} is {@code null}.
*/
public void beginExport(File file) throws IOException;
/**
* Must be invoked at beginning of export to an {@link OutputStream}.
*
* The used {@link Charset} is the one defined in {@link WorkbookWriterFeatures}
* associated to the {@link ImpExFactoryFeatures}.
*
* @param out The output stream.
* @param format The format.
* @throws IOException When a IO error occurs.
* @throws IllegalArgumentException When {@code out} or {@code format} is {@code null},
* or when {@code format} is not supported.
*/
public void beginExport(OutputStream out,
ImpExFormat format) throws IOException;
public void addReadme(List templateInstances) throws IOException;
/**
* Must be invoked at the beginning of a sheet export.
*
* Using several times the same template in a workbook must be supported.
*
* @param templateInstance The sheet template instance used for that sheet.
* @param sheetName The sheet name.
* @param numberOfRows The number of rows to export in this sheet, or -1L if unknown.
* @throws IOException When a IO error occurs.
*/
public void beginSheet(SheetTemplateInstance templateInstance,
String sheetName,
long numberOfRows) throws IOException;
public void addRow(ExportRow row) throws IOException;
/**
* Must be invoked at the end of writing a sheet.
*
* @throws IOException When a IO error occurs.
*/
public void endSheet() throws IOException;
/**
* Must be invoked at end of export.
*
* WARNING This will not close this export driver.
*
* @throws IOException When a IO error occurs.
*/
public void endExport() throws IOException;
}