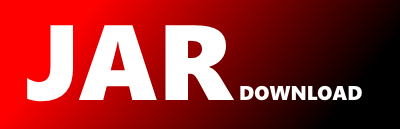
cdc.impex.exports.WorkbookExporter Maven / Gradle / Ivy
package cdc.impex.exports;
import java.util.Map;
import java.util.function.Function;
import cdc.impex.templates.SheetTemplateInstance;
import cdc.issues.Issue;
import cdc.issues.IssuesHandler;
import cdc.util.lang.Checks;
/**
* Interface implemented by classes that can extract workbook data to export.
*
* @author Damien Carbonne
*/
public interface WorkbookExporter extends SheetExporter {
public static final WorkbookExporter QUIET_VOID = new CheckedWorkbookExporter();
public static final WorkbookExporter VERBOSE_VOID = new VerboseWorkbookExporter(QUIET_VOID);
/**
* Invoked to notify the beginning of export.
*
* @param issuesHandler The issues handler that should be used by the application to
* generate new issues.
*/
public default void beginExport(IssuesHandler issuesHandler) {
// Ignore
}
/**
* Invoked to notify the end of export.
*
* @param issuesHandler The issues handler that should be used by the application to
* generate new issues.
*/
public default void endExport(IssuesHandler issuesHandler) {
// Ignore
}
/**
* Creates a delegating workbook exporter.
*
* When the export of a sheet starts, an appropriate sheet exporter is selected,
* if possible, among {@code delegates}.
* If no appropriate sheet exporter is found, the default workbook exporter is used. The default will usually ignore things.
*
* @param def The default workbook exporter.
* It can be {@link #QUIET_VOID}, {@link #VERBOSE_VOID},
* or any other valid WorkbookExporter.
* @param function The function that maps template names to the associated sheet exporters.
* @return A new WorkbookExporter that delegates export if possible, or use the default workbook exporter otherwise.
*/
public static WorkbookExporter fromDelegates(WorkbookExporter def,
Function function) {
Checks.isNotNull(def, "def");
Checks.isNotNull(function, "function");
return new WorkbookExporter() {
private SheetExporter delegate = null;
private SheetExporter get() {
return delegate == null ? def : delegate;
}
@Override
public void beginExport(IssuesHandler issuesHandler) {
def.beginExport(issuesHandler);
}
@Override
public void beginSheetExport(SheetTemplateInstance templateInstance,
IssuesHandler issuesHandler) {
delegate = function.apply(templateInstance.getTemplate().getName());
get().beginSheetExport(templateInstance, issuesHandler);
}
@Override
public int getNumberOfRemainingRows() {
return get().getNumberOfRemainingRows();
}
@Override
public void exportRow(ExportRow row,
IssuesHandler issuesHandler) {
get().exportRow(row, issuesHandler);
}
@Override
public void endSheetExport(SheetTemplateInstance templateInstance,
IssuesHandler issuesHandler) {
get().endSheetExport(templateInstance, issuesHandler);
}
@Override
public void endExport(IssuesHandler issuesHandler) {
def.endExport(issuesHandler);
}
};
}
public static WorkbookExporter fromDelegates(Function function) {
return fromDelegates(QUIET_VOID, function);
}
/**
* Creates a delegating workbook exporter.
*
* @param def The default workbook exporter.
* It can be {@link #QUIET_VOID}, {@link #VERBOSE_VOID},
* or any other valid WorkbookExporter.
* @param map The map from template names to the associated sheet exporters.
* @return A new WorkbookExporter that delegates export if possible, or use the default workbook exporter otherwise.
*/
public static WorkbookExporter fromDelegates(WorkbookExporter def,
Map map) {
return fromDelegates(def, map::get);
}
public static WorkbookExporter fromDelegates(Map map) {
return fromDelegates(map::get);
}
}