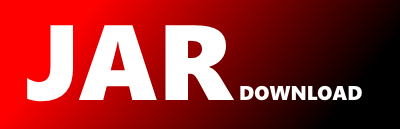
cdc.impex.imports.ImportRow Maven / Gradle / Ivy
package cdc.impex.imports;
import java.util.List;
import java.util.Optional;
import java.util.Set;
import cdc.impex.ImpExNames;
import cdc.impex.templates.ColumnTemplate;
import cdc.impex.templates.ImportAction;
import cdc.impex.templates.SheetTemplate;
import cdc.impex.templates.SheetTemplateInstance;
import cdc.issues.Issue;
import cdc.issues.locations.WorkbookLocation;
import cdc.util.lang.Checks;
/**
* Definition of an import row.
*
* At the raw level, it is an (actual name, string value) map.
* At the typed level, it is an (actual name, object value) map.
*
* @author Damien Carbonne
*/
public interface ImportRow {
/**
* The special value used to erase (set to null) an attribute.
*
* This special value can only be used for updates of optional data.
*/
public static final String ERASE = "ERASE";
/**
* @return The systemId of the imported data.
*/
public String getSystemId();
/**
* @return The sheet name of the imported row.
*/
public String getSheetName();
public SheetTemplateInstance getTemplateInstance();
/**
* @return The associated template.
*/
public SheetTemplate getTemplate();
/**
* @return The column names.
*/
public Set getNames();
/**
* Returns the number (1-based) of this row.
*
* The first data row should start at 2.
*
* @return The row number (1-based).
*/
public int getNumber();
/**
* @return The action to be taken for this row.
* @deprecated Use {@link #getAction(Optional)}.
*/
@Deprecated(since = "2024-06-29", forRemoval = true)
public default ImportAction getAction() {
return getAction(Optional.empty());
}
/**
* Returns the action to take for the row:
*
* - If an action is defined in the row, then it is returned.
*
- If no action is defined in the row, and {@code defaultAction} is set, then {@code defaultAction} is returned.
*
- If no action is defined in the row, and {@code defaultAction} is not set, then {@link SheetTemplate#getDefaultAction()}
* is returned.
*
*
* @param defaultAction The optional default action.
* @return The action to be taken for this row.
*/
public ImportAction getAction(Optional defaultAction);
/**
* Returns {@code true} if the data associated to a column must be erased.
*
* @param name The column name.
* @return {@code true} if the data associated to column named {@code name} must be erased.
* @throws IllegalArgumentException When {@code name} is {@code null}.
*/
public boolean isErase(String name);
/**
* Returns {@code true} if the data associated to a name column must be erased.
*
* WARNING: MUST be used with a name column.
*
* @param column The column.
* @return {@code true} if the data associated to {@code column} must be erased.
* @throws IllegalArgumentException When {@code column} is {@code null} or is not a name column.
*/
public default boolean isErase(ColumnTemplate> column) {
Checks.isNotNull(column, ImpExNames.COLUMN);
column.checkIsName();
return isErase(column.getName());
}
/**
* Returns the raw data associated to a column or {@code null}.
*
* @param name The column name.
* @return The raw data associated to column named {@code name} or {@code null}.
* @throws IllegalArgumentException When {@code name} is {@code null}.
*/
public String getRawDataOrNull(String name);
/**
* Returns the data associated to a column or {@code null}.
*
* If data must be erased, {@code null} is returned.
*
* @param name The column name.
* @return The data associated to column named {@code name} or {@code null}.
* @throws IllegalArgumentException When {@code name} is {@code null}.
*/
public Object getDataOrNull(String name);
/**
* Returns the data associated to a column or a default value.
*
* If data must be erased, the default value {@code def} is returned.
*
* @param name The column name.
* @param def The default value.
* @return The data associated to column named {@code name} or {@code def}.
* @throws IllegalArgumentException When {@code name} is {@code null}.
*/
public default Object getData(String name,
Object def) {
Checks.isNotNull(name, ImpExNames.NAME);
final Object data = getDataOrNull(name);
return data == null ? def : data;
}
/**
* Returns the data associated to a column or {@code null}.
*
* WARNING: if data must be erased, {@code null} is returned.
*
* @param The result type.
* @param cls The result class.
* @param name The column name.
* @return The data associated to column named {@code name} or {@code null}.
* @throws IllegalArgumentException When {@code cls} or {@code name} is {@code null}.
* @throws ClassCastException When the associated data can not be converted to {@code cls}
*/
public T getDataOrNull(Class cls,
String name);
/**
* Returns the data associated to a column or a default value.
*
* WARNING: if data must be erased, the default value ({@code def}) is returned.
*
* @param The result type.
* @param cls The result class.
* @param name The column name.
* @param def The default value.
* @return The data associated to column named {@code name} or {@code def}.
* @throws IllegalArgumentException When {@code cls} or {@code name} is {@code null}.
* @throws ClassCastException When the associated data can not be converted to {@code cls}
*/
public default T getData(Class cls,
String name,
T def) {
Checks.isNotNull(cls, ImpExNames.CLS);
Checks.isNotNull(name, ImpExNames.NAME);
final T data = getDataOrNull(cls, name);
return data == null ? def : data;
}
/**
* Returns the data associated to a column or {@code null}.
*
* WARNING: if data must be erased, {@code null} is returned.
*
* @param The result type.
* @param column The column.
* @return The data associated to {@code column} or {@code null}.
* @throws IllegalArgumentException When {@code column} is {@code null} or is not a name column.
* @throws ClassCastException When the associated data can not be converted to {@code column} data type.
*/
public default T getDataOrNull(ColumnTemplate column) {
Checks.isNotNull(column, ImpExNames.COLUMN);
column.checkIsName();
return getDataOrNull(column.getDataType(),
column.getName());
}
/**
* Returns the data associated to a column or a default value.
*
* WARNING: if data must be erased, the default value {@code def} is returned.
*
* @param The result type.
* @param column The column.
* @param def The default value.
* @return The data associated to {@code column} or {@code def}.
* @throws IllegalArgumentException When {@code column} is {@code null} or is not a name.
* @throws ClassCastException When the associated data can not be converted to {@code column} data type.
*/
public default T getData(ColumnTemplate column,
T def) {
Checks.isNotNull(column, ImpExNames.COLUMN);
final T data = getDataOrNull(column);
return data == null ? def : data;
}
/**
* Returns the data associated to a column or the column default value.
*
* WARNING: if data must be erased, the column default value is returned.
*
* @param The result type.
* @param column The column.
* @return The data associated to {@code column} or the column default value ({@link ColumnTemplate#getDef()}).
*/
public default T getDataOrDef(ColumnTemplate column) {
Checks.isNotNull(column, ImpExNames.COLUMN);
column.checkIsName();
return getData(column.getDataType(),
column.getName(),
column.getDef());
}
/**
* @return The issues associated to this row.
*/
public List getIssues();
/**
* @return {@code true} if this row can be processed.
* There are no CRITICAL (or more severe) issues.
*/
public boolean canBeProcessed();
/**
* @return {@code true} if this row is empty.
* This may happen when rows are removed from a sheet.
*/
public boolean isEmpty();
/**
* @return The location corresponding to this row.
*/
public WorkbookLocation getLocation();
}