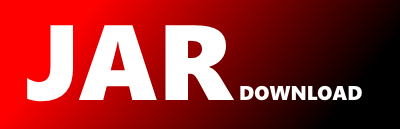
cdc.impex.imports.SheetImporter Maven / Gradle / Ivy
package cdc.impex.imports;
import java.util.Map;
import java.util.function.Function;
import cdc.impex.templates.SheetTemplate;
import cdc.impex.templates.SheetTemplateInstance;
import cdc.issues.Issue;
import cdc.issues.IssuesHandler;
/**
* Interface implemented by classes that can import data from memory to storage device.
*
* [File] → {@link Importer} → [Memory] → {@link SheetImporter} → [Storage Device].
*
* Typically, it will consist in executing actions and propagate them to a database.
*
* An implementation can support one one several templates.
* As {@link Importer} uses one {@link WorkbookImporter}, use, for example,
* {@link WorkbookImporter#fromDelegates(WorkbookImporter, Function)}
* or {@link WorkbookImporter#fromDelegates(WorkbookImporter, Map)} to compose several SheetImporters into one.
*
* @author Damien Carbonne
*/
public interface SheetImporter {
public static final SheetImporter QUIET_VOID = new CheckedSheetImporter();
public static final SheetImporter VERBOSE_VOID = new VerboseSheetImporter(QUIET_VOID);
/**
* Invoked to notify the beginning of the import of a sheet.
*
* @param systemId The system if of the imported data.
* @param sheetName The sheet name.
* @param template The sheet template associated to this import.
* @param issuesHandler The issues handler that should be used by the application to
* generate new issues.
*/
public default void beginSheetImport(String systemId,
String sheetName,
SheetTemplate template,
IssuesHandler issuesHandler) {
// Ignore
}
public default void importHeader(SheetTemplateInstance templateInstance,
IssuesHandler issuesHandler) {
// Ignore
}
/**
* Invoked to notify the import a row.
*
* @param row The imported row.
* @param issuesHandler The issues handler that should be used by the application to
* generate new issues.
*/
public void importRow(ImportRow row,
IssuesHandler issuesHandler);
/**
* Invoked to notify the end of the import of a sheet.
*
* @param systemId The system if of the imported data.
* @param sheetName The sheet name.
* @param templateInstance The sheet template instance associated to this import.
* @param issuesHandler The issues handler that should be used by the application to
* generate new issues.
*/
public default void endSheetImport(String systemId,
String sheetName,
SheetTemplateInstance templateInstance,
IssuesHandler issuesHandler) {
// Ignore
}
}