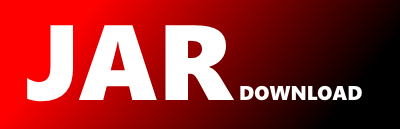
cdc.impex.tools.ImpExToolbox Maven / Gradle / Ivy
package cdc.impex.tools;
import java.io.File;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import java.util.Set;
import javax.swing.SwingUtilities;
import javax.swing.WindowConstants;
import org.apache.commons.cli.CommandLine;
import org.apache.commons.cli.Option;
import org.apache.commons.cli.Options;
import org.apache.commons.cli.ParseException;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import cdc.impex.ImpExFactory;
import cdc.impex.ImpExFactoryFeatures;
import cdc.impex.imports.Importer;
import cdc.impex.imports.WorkbookImporter;
import cdc.impex.templates.SheetTemplate;
import cdc.impex.templates.TemplateGenerator;
import cdc.impex.tools.ImpExToolbox.MainArgs.Feature;
import cdc.impex.tools.swing.ImpExToolboxFrame;
import cdc.issues.Issue;
import cdc.issues.IssuesCollector;
import cdc.issues.impl.IssuesAndAnswersImpl;
import cdc.issues.io.IssuesIoFactoryFeatures;
import cdc.issues.io.IssuesWriter;
import cdc.issues.io.OutSettings;
import cdc.util.cli.AbstractMainSupport;
import cdc.util.cli.FeatureMask;
import cdc.util.cli.MainResult;
import cdc.util.cli.OptionEnum;
import cdc.util.events.ProgressController;
import cdc.util.files.Files;
import cdc.util.lang.FailureReaction;
import cdc.util.lang.Introspection;
public final class ImpExToolbox {
private static final Logger LOGGER = LogManager.getLogger(ImpExToolbox.class);
private final MainArgs margs;
private ImpExToolbox(MainArgs margs) {
this.margs = margs;
}
public static class MainArgs {
public File outputDir;
public String prefix;
public final List preload = new ArrayList<>();
public final List templates = new ArrayList<>();
public final FeatureMask features = new FeatureMask<>();
public File templateFile;
public List filesToCheck = new ArrayList<>();
public File issuesFile;
public enum Feature implements OptionEnum {
ALL_TEMPLATES("all-templates", "If enabled, use or select all templates."),
CLI("cli", "If enabled, run as CLI."),
GUI("gui", "If enabled, run as GUI (default).");
private final String name;
private final String description;
private Feature(String name,
String description) {
this.name = name;
this.description = description;
}
@Override
public final String getName() {
return name;
}
@Override
public final String getDescription() {
return description;
}
}
}
private void execute() {
for (final String name : margs.preload) {
Introspection.getClass(name, true, FailureReaction.WARN);
}
if (!margs.features.contains(Feature.CLI)) {
SwingUtilities.invokeLater(() -> {
final ImpExToolboxFrame frame = new ImpExToolboxFrame(margs);
frame.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
frame.setVisible(true);
});
} else {
generateTemplateFile();
checkFiles();
}
}
private void generateTemplateFile() {
if (margs.templateFile != null) {
final List templates;
if (margs.features.isEnabled(Feature.ALL_TEMPLATES)) {
templates = new ArrayList<>(Settings.CATALOG.getTemplates());
templates.sort(SheetTemplate.DOMAIN_NAME_COMPARATOR);
} else {
templates = Settings.CATALOG.getTemplatesAsList(margs.templates);
}
final ImpExFactory factory = new ImpExFactory(ImpExFactoryFeatures.BEST);
final File file = margs.templateFile;
final TemplateGenerator generator = factory.createTemplateGenerator(file);
try {
LOGGER.info("Generate {}", file);
generator.generate(file, templates);
LOGGER.info("Generated {}", file);
} catch (final IOException e) {
LOGGER.catching(e);
}
}
}
private void checkFiles() {
if (!margs.filesToCheck.isEmpty()) {
final ImpExFactory factory = new ImpExFactory(ImpExFactoryFeatures.FASTEST);
final Set templates;
if (margs.features.isEnabled(Feature.ALL_TEMPLATES)) {
templates = Settings.CATALOG.getTemplates();
} else {
templates = Settings.CATALOG.getTemplatesAsSet(margs.templates);
}
final IssuesCollector issuesCollector = new IssuesCollector<>();
for (final File file : margs.filesToCheck) {
final Importer importer = factory.createImporter(file);
try {
importer.importData(file,
templates,
WorkbookImporter.QUIET_VOID,
issuesCollector,
ProgressController.VOID);
} catch (final IOException e) {
LOGGER.catching(e);
}
}
try {
IssuesWriter.save(new IssuesAndAnswersImpl().addIssues(issuesCollector.getIssues()),
OutSettings.ALL_DATA_ANSWERS,
margs.issuesFile,
ProgressController.VOID,
IssuesIoFactoryFeatures.UTC_BEST);
} catch (final IOException e) {
LOGGER.catching(e);
}
}
}
public static void execute(MainArgs margs) {
final ImpExToolbox instance = new ImpExToolbox(margs);
instance.execute();
}
public static MainResult exec(String... args) {
final MainSupport support = new MainSupport();
support.main(args);
return support.getResult();
}
public static void main(String... args) {
final int code = exec(args).getCode();
System.exit(code);
}
private static class MainSupport extends AbstractMainSupport {
private static final String PREFIX = "prefix";
private static final String PRELOAD = "preload";
private static final String TEMPLATE = "template";
private static final String GENERATE_TEMPLATE = "generate-template";
private static final String CHECK_FILE = "check-file";
private static final String ISSUES_FILE = "issues-file";
public MainSupport() {
super(ImpExToolbox.class, LOGGER);
}
@Override
protected String getVersion() {
return Config.VERSION;
}
@Override
protected void addSpecificOptions(Options options) {
options.addOption(Option.builder()
.longOpt(OUTPUT_DIR)
.desc("Name of the output directory.")
.hasArg()
.build());
options.addOption(Option.builder()
.longOpt(PREFIX)
.desc("Prefix to use for generated files. GUI mode.")
.hasArg()
.build());
options.addOption(Option.builder()
.longOpt(PRELOAD)
.desc("Names of class(es) to preload.\nThose classes should statically initialize Settings.CATALOG to declare templates that should be used.")
.hasArgs()
.build());
options.addOption(Option.builder()
.longOpt(TEMPLATE)
.desc("Name(s) of template(s) to use or select.")
.hasArgs()
.build());
options.addOption(Option.builder()
.longOpt(GENERATE_TEMPLATE)
.desc("Name of the template file to generate. CLI mode.")
.hasArg()
.build());
options.addOption(Option.builder()
.longOpt(CHECK_FILE)
.desc("Name(s) of the file(s) to check. CLI mode.")
.hasArgs()
.build());
options.addOption(Option.builder()
.longOpt(ISSUES_FILE)
.desc("Name of the issues file. CLI mode.")
.hasArg()
.build());
addNoArgOptions(options, MainArgs.Feature.class);
createGroup(options,
MainArgs.Feature.CLI,
MainArgs.Feature.GUI);
}
@Override
protected MainArgs analyze(CommandLine cl) throws ParseException {
final MainArgs margs = new MainArgs();
margs.outputDir = getValueAsFile(cl, OUTPUT_DIR, Files.currentDir(), IS_DIRECTORY);
margs.prefix = getValueAsString(cl, PREFIX, "impex");
margs.preload.addAll(getValues(cl, PRELOAD));
margs.templates.addAll(getValues(cl, TEMPLATE));
margs.templateFile = getValueAsFile(cl, GENERATE_TEMPLATE);
margs.filesToCheck.addAll(getValues(cl, CHECK_FILE, File::new));
margs.issuesFile = getValueAsFile(cl, ISSUES_FILE, IS_NULL_OR_FILE);
setMask(cl, MainArgs.Feature.class, margs.features::setEnabled);
if (!margs.filesToCheck.isEmpty() && margs.issuesFile == null) {
throw new ParseException(ISSUES_FILE + " is missing.");
}
for (final File file : margs.filesToCheck) {
if (!file.isFile()) {
throw new ParseException(file + " is not an existing file.");
}
}
return margs;
}
@Override
protected Void execute(MainArgs margs) {
ImpExToolbox.execute(margs);
return null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy