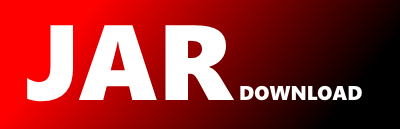
cdc.impex.tools.swing.SheetTemplateViewer Maven / Gradle / Ivy
package cdc.impex.tools.swing;
import java.awt.Color;
import java.awt.Font;
import java.awt.GridBagConstraints;
import java.awt.GridBagLayout;
import javax.swing.BorderFactory;
import javax.swing.JComponent;
import javax.swing.JPanel;
import javax.swing.JTextArea;
import javax.swing.border.CompoundBorder;
import cdc.impex.templates.ColumnTemplate;
import cdc.impex.templates.SheetTemplate;
import cdc.ui.swing.GridBagConstraintsBuilder;
import cdc.validation.checkers.Checker;
/**
* Panel used to display a SheetTemaplate.
*
* @author Damien Carbonne
*/
public class SheetTemplateViewer extends JPanel {
private static final long serialVersionUID = 1L;
private SheetTemplate template = null;
private static final Color HEADER_COLOR = new Color(0.85F, 0.85F, 0.85F);
public SheetTemplateViewer() {
setLayout(new GridBagLayout());
}
private static JComponent create(String text,
boolean header,
boolean dim) {
final JTextArea w = new JTextArea();
w.setBorder(new CompoundBorder(BorderFactory.createLineBorder(Color.BLACK),
BorderFactory.createEmptyBorder(2, 2, 2, 2)));
w.setText(text);
w.setEditable(false);
if (header) {
w.setBackground(HEADER_COLOR);
final Font font = w.getFont();
w.setFont(font.deriveFont(Font.BOLD, font.getSize() * 1.3F));
w.setColumns(15);
}
if (dim) {
w.setLineWrap(true);
w.setWrapStyleWord(true);
}
return w;
}
public void setTemplate(SheetTemplate template) {
removeAll();
this.template = template;
if (template != null) {
// Template QName
add(create(template.getQName(), true, false),
GridBagConstraintsBuilder.builder()
.gridx(0)
.gridy(0)
.fill(GridBagConstraints.BOTH)
.build());
// Template Description
add(create(template.getDescription(), false, true),
GridBagConstraintsBuilder.builder()
.gridx(0)
.gridy(1)
.gridheight(3)
.fill(GridBagConstraints.BOTH)
.build());
int x = 1;
for (final ColumnTemplate> column : template.getColumns()) {
// Column name
add(create(column.getName(), true, false),
GridBagConstraintsBuilder.builder()
.gridx(x)
.gridy(0)
.fill(GridBagConstraints.BOTH)
.build());
// Column data type and usage
add(create(column.getUsage() + " " + column.getDataType().getSimpleName(), false, false),
GridBagConstraintsBuilder.builder()
.gridx(x)
.gridy(1)
.fill(GridBagConstraints.BOTH)
.build());
// Column description
add(create(column.getDescription(), false, true),
GridBagConstraintsBuilder.builder()
.gridx(x)
.gridy(2)
.fill(GridBagConstraints.BOTH)
.build());
// Column checker
final Checker> checker = column.getCheckerOrNull();
add(create(checker == null ? "" : checker.explain(), false, true),
GridBagConstraintsBuilder.builder()
.gridx(x)
.gridy(3)
.fill(GridBagConstraints.BOTH)
.build());
x++;
}
}
revalidate();
repaint();
}
public SheetTemplate getTemplate() {
return template;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy