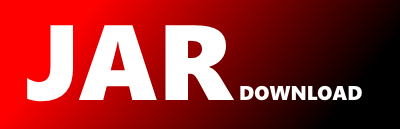
cdc.impex.tools.swing.SheetTemplatesPanel Maven / Gradle / Ivy
package cdc.impex.tools.swing;
import java.awt.Dimension;
import java.awt.FlowLayout;
import java.awt.GridBagConstraints;
import java.awt.GridBagLayout;
import javax.swing.JButton;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.ListSelectionModel;
import cdc.impex.tools.ImpExToolbox.MainArgs.Feature;
import cdc.ui.swing.GridBagConstraintsBuilder;
import cdc.ui.swing.SwingUtils;
/**
* Sub-panel used to select templates to use.
*
* @author Damien Carbonne
*/
class SheetTemplatesPanel extends JPanel {
private static final long serialVersionUID = 1L;
private final ImpExToolboxFrame wFrame;
private final JTable wTable;
private final SheetTemplatesTableModel model = new SheetTemplatesTableModel();
private final JButton wSelectAll = new JButton("All");
private final JButton wDeselectAll = new JButton("None");
public SheetTemplatesPanel(ImpExToolboxFrame frame) {
this.wFrame = frame;
setLayout(new GridBagLayout());
final JScrollPane wScrollPane = new JScrollPane();
add(wScrollPane,
GridBagConstraintsBuilder.builder()
.gridx(0)
.gridy(0)
.weightx(1.0)
.weighty(1.0)
.fill(GridBagConstraints.BOTH)
.build());
wScrollPane.setPreferredSize(new Dimension(600, 200));
wTable = new JTable(model);
wTable.setAutoCreateRowSorter(true);
wTable.setSelectionMode(ListSelectionModel.SINGLE_SELECTION);
wTable.setAutoResizeMode(JTable.AUTO_RESIZE_OFF);
SwingUtils.setColumnWidths(wTable, 75, 150, 250);
wScrollPane.setViewportView(wTable);
final JPanel wControls = new JPanel();
wControls.setLayout(new FlowLayout(FlowLayout.TRAILING));
add(wControls,
GridBagConstraintsBuilder.builder()
.gridx(0)
.gridy(1)
.weightx(1.0)
.weighty(0.0)
.fill(GridBagConstraints.BOTH)
.build());
wControls.add(wSelectAll);
wControls.add(wDeselectAll);
wSelectAll.addActionListener(e -> model.selectAll());
wDeselectAll.addActionListener(e -> model.deselectAll());
if (wFrame.margs.features.isEnabled(Feature.ALL_TEMPLATES)) {
model.selectAll();
} else {
for (final String name : wFrame.margs.templates) {
model.select(name);
}
}
}
public SheetTemplatesTableModel getModel() {
return model;
}
public JTable getTable() {
return wTable;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy