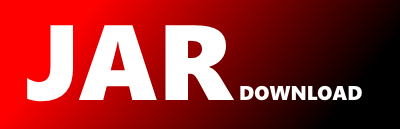
cdc.io.benches.XmlDataPerf Maven / Gradle / Ivy
package cdc.io.benches;
import java.io.BufferedInputStream;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.io.OutputStreamWriter;
import java.io.Writer;
import java.nio.charset.StandardCharsets;
import javax.xml.parsers.ParserConfigurationException;
import javax.xml.parsers.SAXParser;
import javax.xml.parsers.SAXParserFactory;
import org.xml.sax.InputSource;
import org.xml.sax.SAXException;
import org.xml.sax.ext.DefaultHandler2;
import cdc.io.data.Document;
import cdc.io.data.Element;
import cdc.io.data.NodeType;
import cdc.io.data.util.DataStats;
import cdc.io.data.xml.XmlDataReader;
import cdc.io.data.xml.XmlDataWriter;
import cdc.io.xml.XmlWriter;
import cdc.io.xml.XmlWriter.Feature;
import cdc.util.lang.BlackHole;
import cdc.util.time.Chronometer;
public final class XmlDataPerf {
private static final String ROOT = "root";
private static final String CHILD = "child";
private static final String INDENT = " ";
private XmlDataPerf() {
}
private static Document generateDocument(int count) {
final Document doc = new Document();
final Element root = new Element(doc, ROOT);
for (int index = 0; index < count; index++) {
root.addElement(CHILD);
}
return doc;
}
private static void saveDocument(Document doc,
File file,
XmlWriter.Feature... features) throws IOException {
XmlDataWriter.save(doc, file, INDENT, features);
}
private static void writeToFile(File file,
int count) throws IOException {
try (final XmlWriter writer = new XmlWriter(file)) {
if (INDENT != null) {
writer.setEnabled(Feature.PRETTY_PRINT);
}
writer.setIndentString(INDENT);
writer.beginDocument();
writer.beginElement(ROOT);
for (int index = 0; index < count; index++) {
writer.beginElement(CHILD);
writer.endElement();
}
writer.endElement();
writer.endDocument();
writer.flush();
}
}
private static void writeToMemory(int count) throws IOException {
try (final XmlWriter writer = new XmlWriter(new ByteArrayOutputStream())) {
if (INDENT != null) {
writer.setEnabled(Feature.PRETTY_PRINT);
}
writer.setIndentString(INDENT);
writer.beginDocument();
writer.beginElement(ROOT);
for (int index = 0; index < count; index++) {
writer.beginElement(CHILD);
writer.endElement();
}
writer.endElement();
writer.endDocument();
writer.flush();
}
}
private static void rawWriteToMemory1(int count) throws IOException {
final OutputStream os = new ByteArrayOutputStream(count);
try (final Writer writer = new OutputStreamWriter(os, StandardCharsets.UTF_8)) {
writer.append("\n");
writer.append("<");
writer.append(ROOT);
writer.append(">\n");
for (int index = 0; index < count; index++) {
writer.append(INDENT);
writer.append("<");
writer.append(CHILD);
writer.append("/>\n");
}
writer.append("");
writer.append(ROOT);
writer.append(">");
writer.flush();
}
}
private static void rawWriteToMemory2(int count) throws IOException {
final OutputStream os = new ByteArrayOutputStream(count);
try (final Writer writer = new OutputStreamWriter(os, StandardCharsets.UTF_8)) {
writer.append("\n");
writer.append("\n");
for (int index = 0; index < count; index++) {
writer.append(" \n");
}
writer.append(" ");
writer.flush();
}
}
private static Document load(File file) throws IOException {
final XmlDataReader reader = new XmlDataReader();
final Document doc = reader.read(file);
return doc;
}
private static class Handler extends DefaultHandler2 {
public Handler() {
super();
}
}
private static void parse1(File file) throws IOException {
try {
final SAXParserFactory factory = SAXParserFactory.newInstance();
final Handler handler = new Handler();
final SAXParser parser = factory.newSAXParser();
final InputStream is = new BufferedInputStream(new FileInputStream(file));
final InputSource source = new InputSource(is);
source.setSystemId(file.getPath());
parser.parse(source, handler);
} catch (final SAXException | ParserConfigurationException e) {
throw new IOException(e);
}
}
private static void parse2(File file) throws IOException {
try {
final SAXParserFactory factory = SAXParserFactory.newInstance();
final Handler handler = new Handler();
final SAXParser parser = factory.newSAXParser();
final InputStream is = new FileInputStream(file);
final InputSource source = new InputSource(is);
source.setSystemId(file.getPath());
parser.parse(source, handler);
} catch (final SAXException | ParserConfigurationException e) {
throw new IOException(e);
}
}
@SuppressWarnings("unused")
private static void read1(File file) throws IOException {
try (final InputStream is = new FileInputStream(file)) {
int i;
while ((i = is.read()) > 0) {
// Ignore
}
}
}
private static void read2(File file) throws IOException {
try (final InputStream is = new BufferedInputStream((new FileInputStream(file)))) {
@SuppressWarnings("unused")
int i;
while ((i = is.read()) > 0) {
// Ignore
}
}
}
// private static void display(Document doc,
// double seconds) {
// final int count = doc.getRootElement().getChildrenCount();
// System.out.println(count + " " + (int) (count / seconds) + " element/second");
// }
private static void print(String message,
int count,
Chronometer chrono) {
System.out.println(String.format("%40s %12s %,11d e/s",
message,
chrono.toString(),
(int) (count / chrono.getElapsedSeconds())));
}
private static void test1() throws IOException {
final Chronometer chrono = new Chronometer();
final File file = new File("target/test.xml");
for (int k = 0; k < 24; k++) {
for (int l = 0; l < 5; l++) {
final int count = (int) Math.round(Math.pow(2, k));
System.out.println("-----------------------------------------");
System.out.println(String.format("count: %,d", count));
chrono.start();
final Document doc1 = generateDocument(count);
chrono.suspend();
print("generate: ", count, chrono);
chrono.start();
saveDocument(doc1, file);
chrono.suspend();
print("save no features: ", count, chrono);
chrono.start();
saveDocument(doc1, file, XmlWriter.Feature.DONT_VALIDATE_NAMES);
chrono.suspend();
print("save no name validation: ", count, chrono);
chrono.start();
saveDocument(doc1, file, XmlWriter.Feature.DONT_VALIDATE_NAMES, XmlWriter.Feature.DONT_VALIDATE_CHARS);
chrono.suspend();
print("save no name and chars validation: ", count, chrono);
chrono.start();
parse1(file);
chrono.suspend();
print("parse1: ", count, chrono);
chrono.start();
parse2(file);
chrono.suspend();
print("parse2: ", count, chrono);
// chrono.start();
// read1(file);
// chrono.suspend();
// print("read1: ", count, chrono);
chrono.start();
read2(file);
chrono.suspend();
print("read2: ", count, chrono);
file.delete();
chrono.start();
writeToFile(file, count);
chrono.suspend();
print("xml file: ", count, chrono);
chrono.start();
writeToMemory(count);
chrono.suspend();
print("xml mem: ", count, chrono);
chrono.start();
rawWriteToMemory1(count);
chrono.suspend();
print("raw mem1: ", count, chrono);
chrono.start();
rawWriteToMemory2(count);
chrono.suspend();
print("raw mem2: ", count, chrono);
chrono.start();
final Document doc = load(file);
BlackHole.discard(doc);
chrono.suspend();
print("load: ", count, chrono);
// display(doc, chrono.getElapsedSeconds());
}
}
}
@SuppressWarnings("unused")
private static void test2() throws IOException {
final int count = 1024 * 1024 * 32;
final File file = new File("target/test.xml");
final Chronometer chrono = new Chronometer();
chrono.start();
writeToFile(file, count);
print("xml file: ", count, chrono);
}
@SuppressWarnings("unused")
private static void test3() throws IOException {
// final int count = 1024 * 1024 * 4;
final File file = new File("/home/damien/z2M.xml");
final Chronometer chrono = new Chronometer();
for (int i = 0; i < 10; i++) {
chrono.start();
final Document doc = load(file);
System.out.println("stats");
final DataStats stats = new DataStats(doc);
stats.print(System.out);
chrono.suspend();
print("load: ", stats.getNodesCount(NodeType.ELEMENT), chrono);
chrono.start();
parse1(file);
chrono.suspend();
print("parse1: ", stats.getNodesCount(NodeType.ELEMENT), chrono);
}
}
public static void main(String[] args) throws IOException {
test1();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy